UG NX8.5,实现OBB算法
时间: 2023-11-10 09:45:08 浏览: 189
UG NX8.5中实现OBB算法需要以下步骤:
1. 创建一个包围盒,将其设置为OBB类型。
2. 将包围盒的方向设置为对象的主方向,并将其位置设置为对象的中心。
3. 计算对象的边界框(AABB)。
4. 将对象的每个顶点变换到包围盒的本地坐标系中。
5. 在包围盒的本地坐标系中计算对象的OBB。
6. 将OBB变换回世界坐标系。
7. 可以使用OBB来进行碰撞检测或者包围盒测试。
这些步骤可以使用UG NX8.5中的API来实现。具体实现细节可以参考UG NX8.5的开发文档或者API文档。
相关问题
UG8.5 NX二次开发 OBB算法获取实体的最小包容盒,C++语言实现
下面是C++语言实现的OBB算法获取实体的最小包容盒的示例代码:
```c++
#include <iostream>
#include <vector>
#include <Eigen/Core>
#include <Eigen/Eigenvalues>
using namespace std;
using namespace Eigen;
Matrix3f computeCovariance(const vector<Vector3f>& points)
{
Vector3f mean = Vector3f::Zero();
for (int i = 0; i < points.size(); ++i) {
mean += points[i];
}
mean /= points.size();
Matrix3f cov = Matrix3f::Zero();
for (int i = 0; i < points.size(); ++i) {
Vector3f p = points[i] - mean;
cov += p * p.transpose();
}
cov /= points.size();
return cov;
}
void computeOBB(const vector<Vector3f>& points, Matrix3f& orientation, Vector3f& center, Vector3f& halfExtents)
{
// Compute covariance matrix
Matrix3f cov = computeCovariance(points);
// Compute eigenvectors and eigenvalues of covariance matrix
SelfAdjointEigenSolver<Matrix3f> eigensolver(cov);
Matrix3f eigenVectors = eigensolver.eigenvectors();
Vector3f eigenValues = eigensolver.eigenvalues();
// Determine orientation of OBB
orientation = eigenVectors;
// Determine center of OBB
center = Vector3f::Zero();
for (int i = 0; i < points.size(); ++i) {
center += points[i];
}
center /= points.size();
center = orientation.transpose() * center;
// Determine half-extents of OBB
halfExtents = Vector3f::Zero();
for (int i = 0; i < points.size(); ++i) {
Vector3f p = orientation.transpose() * (points[i] - center);
halfExtents[0] = max(halfExtents[0], abs(p[0]));
halfExtents[1] = max(halfExtents[1], abs(p[1]));
halfExtents[2] = max(halfExtents[2], abs(p[2]));
}
}
int main()
{
vector<Vector3f> points = { Vector3f(1, 2, 3), Vector3f(4, 5, 6), Vector3f(7, 8, 9) };
Matrix3f orientation;
Vector3f center;
Vector3f halfExtents;
computeOBB(points, orientation, center, halfExtents);
cout << "Orientation:" << endl << orientation << endl;
cout << "Center: " << center.transpose() << endl;
cout << "Half-Extents: " << halfExtents.transpose() << endl;
return 0;
}
```
其中,`computeCovariance`函数用于计算点云的协方差矩阵,`computeOBB`函数用于计算OBB的方向、中心和半轴长度。函数中使用了Eigen库来进行矩阵和向量计算。
UG8.5,在NX二次开发中,用C++结合UG/Open API实现OBB算法获取实体的最小包容盒
UG/Open API提供了获取实体的边界框函数BoundingBox,在此基础上可以实现OBB算法获取实体的最小包容盒。具体实现步骤如下:
1. 首先,通过Open API获取实体的边界框信息。
```cpp
// 获取实体的边界框
tagBoundingBox bBox;
if (!UF_OBJ_ask_bounding_box(entityTag, &bBox))
{
// 获取失败
return false;
}
```
2. 根据边界框信息计算出实体的中心点坐标。
```cpp
// 计算实体的中心点
double centerX = (bBox.min[0] + bBox.max[0]) / 2.0;
double centerY = (bBox.min[1] + bBox.max[1]) / 2.0;
double centerZ = (bBox.min[2] + bBox.max[2]) / 2.0;
```
3. 计算实体在三个坐标轴上的长度、宽度和高度。
```cpp
// 计算实体在三个坐标轴上的长度、宽度和高度
double length = bBox.max[0] - bBox.min[0];
double width = bBox.max[1] - bBox.min[1];
double height = bBox.max[2] - bBox.min[2];
```
4. 将实体的中心点坐标和三个坐标轴上的长度、宽度和高度组合成一个OBB(最小包容盒)。
```cpp
// 组装OBB(最小包容盒)
tagOBB obb;
obb.origin[0] = centerX;
obb.origin[1] = centerY;
obb.origin[2] = centerZ;
obb.xAxis[0] = length / 2.0;
obb.xAxis[1] = 0.0;
obb.xAxis[2] = 0.0;
obb.yAxis[0] = 0.0;
obb.yAxis[1] = width / 2.0;
obb.yAxis[2] = 0.0;
obb.zAxis[0] = 0.0;
obb.zAxis[1] = 0.0;
obb.zAxis[2] = height / 2.0;
```
5. 最后,可以根据需要将OBB转换为其他坐标系下的表示方式,如旋转矩阵和四元数等。
```cpp
// 将OBB转换为旋转矩阵
double rotationMatrix[9];
if (!UF_MTX_build_matrix_from_axes(obb.xAxis, obb.yAxis, obb.zAxis, rotationMatrix))
{
// 转换失败
return false;
}
```
阅读全文
相关推荐
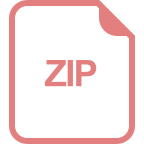
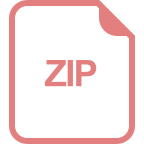
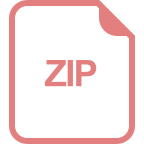








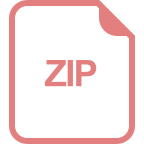
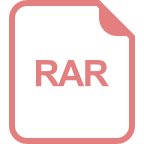
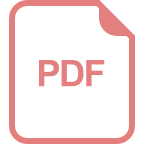
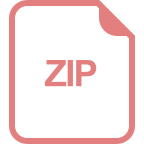
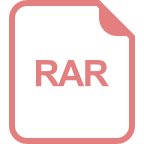