本关任务:编写一个程序,声明抽象基类Shape,由它派生出3个派生类:Circle(圆形)、Rectangle(矩形)、Triangle(三角形),用一个函数printArea分别输出以上三者的面积,3个图形的数据通过键盘依次输入。 相关知识 为了完成本关任务,你需要掌握:多态的概念、虚函数、虚基类。 编程要求 根据提示,在右侧编辑器补充代码。 测试说明 平台会对你编写的代码进行测试: 测试依次输入圆类对象的半径、矩形类对象的宽和高、三角形类对象的底和高:12.6,4.5,8.4,4.5,8.4; 预期输出: area of circle = 498.759 area of rectangle = 37.8 area of triangle = 18.9 开始你的任务吧,祝你成功!
时间: 2024-03-15 11:41:46 浏览: 161
#include <iostream>
using namespace std;
class Shape{
public:
virtual float getArea()=0;
};
class Circle:public Shape{
public:
Circle(float r):radius(r){};
float getArea(){
return 3.14*radius*radius;
}
private:
float radius;
};
class Rectangle:public Shape{
public:
Rectangle(float w,float h):width(w),height(h){};
float getArea(){
return width*height;
}
private:
float width;
float height;
};
class Triangle:public Shape{
public:
Triangle(float b,float h):base(b),height(h){};
float getArea(){
return 0.5*base*height;
}
private:
float base;
float height;
};
void printArea(Shape& shape){
cout<<"area of ";
if(typeid(shape)==typeid(Circle)){
cout<<"circle = ";
}else if(typeid(shape)==typeid(Rectangle)){
cout<<"rectangle = ";
}else if(typeid(shape)==typeid(Triangle)){
cout<<"triangle = ";
}
cout<<shape.getArea()<<endl;
}
int main(){
float r,w,h,b;
cin>>r>>w>>h>>b;
Circle circle(r);
Rectangle rectangle(w,h);
Triangle triangle(b,h);
printArea(circle);
printArea(rectangle);
printArea(triangle);
return 0;
}
阅读全文
相关推荐
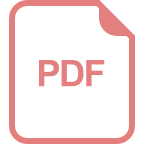
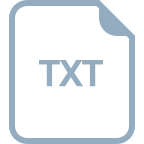
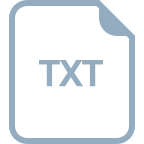















