用c语言编写两个函数,返回一棵给定二叉树在中序遍历下的第一个结点和最后一个结点
时间: 2024-02-22 18:57:48 浏览: 32
好的,下面是两个函数的 C 语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
void first_last_node(struct TreeNode* root, struct TreeNode** first, struct TreeNode** last) {
if (!root) {
*first = *last = NULL;
return;
}
*first = *last = root;
while ((*first)->left) {
*first = (*first)->left;
}
while ((*last)->right) {
*last = (*last)->right;
}
}
void first_last_node_recursive_helper(struct TreeNode* node, struct TreeNode** first, struct TreeNode** last) {
if (!node) {
return;
}
first_last_node_recursive_helper(node->left, first, last);
if (!(*first)) {
*first = node;
}
*last = node;
first_last_node_recursive_helper(node->right, first, last);
}
void first_last_node_recursive(struct TreeNode* root, struct TreeNode** first, struct TreeNode** last) {
*first = *last = NULL;
first_last_node_recursive_helper(root, first, last);
}
```
其中,`first_last_node` 是迭代实现,`first_last_node_recursive` 是递归实现。两个函数的时间复杂度均为 $O(n)$,其中 $n$ 是二叉树的结点数。
需要注意的是,函数的返回值是通过指针参数传递的。上面的代码中,`first` 和 `last` 都是 `struct TreeNode*` 类型的指针,传递进去后,函数内部通过修改指针指向的内存地址来返回值。在调用函数时,需要先声明两个指针变量,然后将它们的地址传递给函数,如下所示:
```c
struct TreeNode* root = ... // 二叉树的根节点
struct TreeNode* first, *last;
first_last_node(root, &first, &last);
printf("First node: %d\n", first->val);
printf("Last node: %d\n", last->val);
```
相关推荐
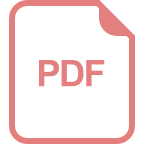
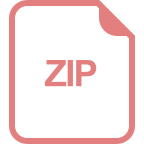














