Java实现一个贪吃蛇小游戏代码并加注释
时间: 2023-06-15 18:03:34 浏览: 88
好的,下面是Java实现贪吃蛇小游戏的代码,并附有详细注释:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.LinkedList;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
// 游戏主体类
public class SnakeGame extends JPanel implements KeyListener {
private static final long serialVersionUID = 1L;
// 游戏区域的大小
private static final int WIDTH = 800;
private static final int HEIGHT = 800;
// 蛇的移动速度
private static final int SPEED = 100;
// 蛇身的长度
private static final int LENGTH = 20;
// 蛇头的初始位置
private static final int HEAD_X = WIDTH / 2;
private static final int HEAD_Y = HEIGHT / 2;
// 食物的初始位置
private static final int FOOD_X = 100;
private static final int FOOD_Y = 100;
// 蛇的移动方向
private static final int DIR_UP = 1;
private static final int DIR_DOWN = -1;
private static final int DIR_LEFT = 2;
private static final int DIR_RIGHT = -2;
// 随机数生成器
private Random random = new Random();
// 记录蛇的身体
private LinkedList<Point> snake = new LinkedList<Point>();
// 记录蛇的移动方向
private int direction = DIR_UP;
// 记录游戏是否结束
private boolean isGameOver = false;
// 记录当前得分
private int score = 0;
// 构造方法
public SnakeGame() {
// 设置游戏区域的大小
setPreferredSize(new java.awt.Dimension(WIDTH, HEIGHT));
// 添加键盘事件监听器
addKeyListener(this);
// 初始化蛇的身体
snake.add(new Point(HEAD_X, HEAD_Y));
snake.add(new Point(HEAD_X, HEAD_Y + LENGTH));
snake.add(new Point(HEAD_X, HEAD_Y + LENGTH * 2));
// 开始游戏循环
new Thread(new Runnable() {
@Override
public void run() {
while (!isGameOver) {
try {
// 暂停一定时间,控制蛇的移动速度
Thread.sleep(SPEED);
// 移动蛇的身体
move();
// 重绘游戏区域
repaint();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}).start();
}
// 绘制游戏区域
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// 绘制蛇的身体
for (Point p : snake) {
g.setColor(Color.GREEN);
g.fillRect(p.x, p.y, LENGTH, LENGTH);
}
// 绘制食物
g.setColor(Color.RED);
g.fillRect(FOOD_X, FOOD_Y, LENGTH, LENGTH);
// 绘制得分
g.setColor(Color.BLACK);
g.drawString("Score: " + score, 10, 20);
// 绘制游戏结束的提示信息
if (isGameOver) {
g.setColor(Color.BLACK);
g.drawString("Game Over", WIDTH / 2 - 30, HEIGHT / 2);
}
}
// 移动蛇的身体
private void move() {
// 计算蛇头的新位置
Point head = snake.getFirst();
Point newHead = new Point(head);
switch (direction) {
case DIR_UP:
newHead.y -= LENGTH;
break;
case DIR_DOWN:
newHead.y += LENGTH;
break;
case DIR_LEFT:
newHead.x -= LENGTH;
break;
case DIR_RIGHT:
newHead.x += LENGTH;
break;
}
// 判断蛇头是否撞到墙壁
if (newHead.x < 0 || newHead.x >= WIDTH || newHead.y < 0 || newHead.y >= HEIGHT) {
isGameOver = true;
return;
}
// 判断蛇头是否撞到自己的身体
for (Point p : snake) {
if (p.equals(newHead)) {
isGameOver = true;
return;
}
}
// 判断蛇头是否吃到了食物
if (newHead.x == FOOD_X && newHead.y == FOOD_Y) {
score += 10;
genFood();
} else {
snake.removeLast();
}
// 将新的蛇头加入到蛇的身体中
snake.addFirst(newHead);
}
// 生成食物的位置
private void genFood() {
int x = random.nextInt(WIDTH / LENGTH) * LENGTH;
int y = random.nextInt(HEIGHT / LENGTH) * LENGTH;
FOOD_X = x;
FOOD_Y = y;
}
// 处理键盘事件,改变蛇的移动方向
public void keyPressed(KeyEvent e) {
switch (e.getKeyCode()) {
case KeyEvent.VK_UP:
if (direction != DIR_DOWN) {
direction = DIR_UP;
}
break;
case KeyEvent.VK_DOWN:
if (direction != DIR_UP) {
direction = DIR_DOWN;
}
break;
case KeyEvent.VK_LEFT:
if (direction != DIR_RIGHT) {
direction = DIR_LEFT;
}
break;
case KeyEvent.VK_RIGHT:
if (direction != DIR_LEFT) {
direction = DIR_RIGHT;
}
break;
}
}
// 必须实现的方法,用于处理键盘事件
public void keyReleased(KeyEvent e) {
}
// 必须实现的方法,用于处理键盘事件
public void keyTyped(KeyEvent e) {
}
// 游戏的入口方法
public static void main(String[] args) {
JFrame frame = new JFrame("贪吃蛇小游戏");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setContentPane(new SnakeGame());
frame.pack();
frame.setVisible(true);
}
}
```
以上就是Java实现贪吃蛇小游戏的代码及注释,希望能对你有所帮助!
相关推荐










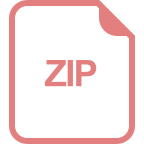
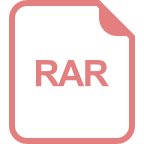
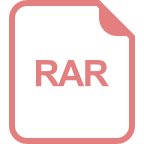
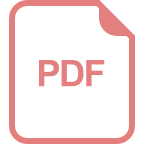