如何通过js实现一个贪吃蛇小游戏,并写出代码和具体中午注释
时间: 2024-06-10 12:03:55 浏览: 7
好的,我会根据你的要求为你提供学习资料和代码示例。首先,你可以参考以下网页,学习如何使用JavaScript编写贪吃蛇小游戏:
1. https://codepen.io/zapplebee/pen/BXyzKv
2. https://www.youtube.com/watch?v=xGmXxpIj6vs
3. https://www.freecodecamp.org/news/how-to-build-a-snake-game-in-javascript/
4. https://www.geeksforgeeks.org/snake-game-in-javascript/
接下来,我为你提供一个示例代码,帮助你实现一个简单的贪吃蛇小游戏。请注意,这只是一个示例代码,你需要根据自己的需要进行修改和优化:
```
<html>
<head>
<title>Snake Game</title>
<style>
canvas {
border: 1px solid black;
}
</style>
</head>
<body>
<canvas id="canvas" width="400" height="400"></canvas>
<script>
// 设置画布和画笔
var canvas = document.getElementById("canvas");
var ctx = canvas.getContext("2d");
// 定义贪吃蛇和食物
var snake = [
{ x: 50, y: 50 },
{ x: 40, y: 50 },
{ x: 30, y: 50 },
{ x: 20, y: 50 }
];
var food = { x: 100, y: 100 };
// 定义贪吃蛇的移动方向
var direction = "right";
// 定义游戏更新函数
function update() {
// 清空画布
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 移动贪吃蛇的头部
var head = { x: snake[0].x, y: snake[0].y };
if (direction == "right") head.x += 10;
else if (direction == "left") head.x -= 10;
else if (direction == "up") head.y -= 10;
else if (direction == "down") head.y += 10;
snake.unshift(head);
// 检查是否吃到了食物
if (head.x === food.x && head.y === food.y) {
food.x = Math.floor(Math.random() * canvas.width / 10) * 10;
food.y = Math.floor(Math.random() * canvas.height / 10) * 10;
} else {
snake.pop();
}
// 绘制贪吃蛇和食物
ctx.fillStyle = "green";
snake.forEach(function (part) {
ctx.fillRect(part.x, part.y, 10, 10);
});
ctx.fillStyle = "red";
ctx.fillRect(food.x, food.y, 10, 10);
// 检查是否撞墙或者撞到自己
if (head.x < 0 || head.x > canvas.width - 10 || head.y < 0 || head.y > canvas.height - 10) {
alert("Game Over!");
location.reload();
}
for (var i = 1; i < snake.length; i++) {
if (head.x === snake[i].x && head.y === snake[i].y) {
alert("Game Over!");
location.reload();
}
}
}
// 定义键盘事件处理函数
window.addEventListener("keydown", function (event) {
if (event.keyCode === 37 && direction !== "right") {
direction = "left";
} else if (event.keyCode === 38 && direction !== "down") {
direction = "up";
} else if (event.keyCode === 39 && direction !== "left") {
direction = "right";
} else if (event.keyCode === 40 && direction !== "up") {
direction = "down";
}
});
// 每100毫秒更新一次游戏
setInterval(update, 100);
</script>
</body>
</html>
```
这段代码实现了一个非常简单的贪吃蛇小游戏,你可以根据需要进行修改和优化。希望能对你有所帮助!
相关推荐
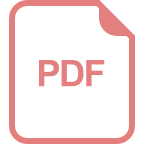
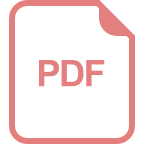
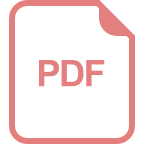














