np.delete函数中axis的作用
时间: 2024-05-14 11:01:28 浏览: 76
`np.delete` 函数可以从一个数组中删除指定的行或列。其中,`axis` 参数用于指定要删除的轴,它的作用如下:
- 当 `axis=None` 时,函数将展开整个数组并删除其中的指定元素。
- 当 `axis=0` 时,函数将删除指定的行。
- 当 `axis=1` 时,函数将删除指定的列。
例如,假设有一个二维数组 `arr`,其形状为 `(3, 4)`,我们想要删除第一行和第三行,可以使用以下代码:
``` python
import numpy as np
arr = np.array([[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]])
new_arr = np.delete(arr, [0, 2], axis=0)
```
其中,`[0, 2]` 表示要删除的行的索引,`axis=0` 表示按行删除。执行完上述代码后,`new_arr` 的值为:
```
array([[ 5, 6, 7, 8],
[ 1, 2, 3, 4]])
```
注意,`np.delete` 函数并不会修改原始数组 `arr`,而是返回一个新的数组 `new_arr`。
相关问题
tensor 中对应np.vstack和np.delete的函数是什么?举例说明
TensorFlow 中对应 np.vstack 的函数是 tf.concat,它可以将多个张量沿着一个新的维度进行拼接。例如,假设有两个形状为 (2, 3) 的张量 A 和 B,那么可以使用 tf.concat([A, B], axis=0) 来将它们在第 0 维进行拼接,得到一个形状为 (4, 3) 的张量。
TensorFlow 中对应 np.delete 的函数是 tf.boolean_mask,它可以根据一个布尔掩码来选择或删除张量中的元素。例如,假设有一个形状为 (3, 4) 的张量 A,那么可以使用 tf.boolean_mask(A, [True, False, True], axis=0) 来选择第 0 和第 2 行,并得到形状为 (2, 4) 的结果。
请问应该在python中导入什么库运行下列代码:def createSpec(data): fs=256 lowcut=117 highcut=123 y=butter_bandstop_filter(data, lowcut, highcut, fs, order=6) lowcut=57 highcut=63 y=butter_bandstop_filter(y, lowcut, highcut, fs, order=6) cutoff=1 y=butter_highpass_filter(y, cutoff, fs, order=6) Pxx=signal.spectrogram(y, nfft=256, fs=256, return_onesided=True, noverlap=128)[2] Pxx = np.delete(Pxx, np.s_[117:123+1], axis=0) Pxx = np.delete(Pxx, np.s_[57:63+1], axis=0) Pxx = np.delete(Pxx, 0, axis=0) result=(10*np.log10(np.transpose(Pxx))-(10*np.log10(np.transpose(Pxx))).min())/(10*np.log10(np.transpose(Pxx))).ptp() return result
您需要导入以下库:
import numpy as np
from scipy import signal
并且需要使用如下代码来定义butter_bandstop_filter和butter_highpass_filter两个函数:
def butter_bandstop_filter(data, lowcut, highcut, fs, order=5):
nyq = 0.5 * fs
low = lowcut / nyq
high = highcut / nyq
b, a = signal.butter(order, [low, high], btype='bandstop')
y = signal.filtfilt(b, a, data)
return y
def butter_highpass_filter(data, cutoff, fs, order=5):
nyq = 0.5 * fs
normal_cutoff = cutoff / nyq
b, a = signal.butter(order, normal_cutoff, btype='highpass')
y = signal.filtfilt(b, a, data)
return y
接下来,您可以直接使用上述代码中的createSpec函数。
阅读全文
相关推荐
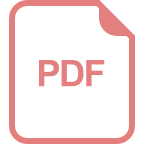
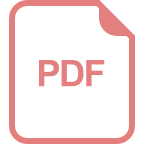
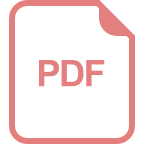













