Spring boot entity mapper impl
时间: 2023-08-14 13:08:22 浏览: 129
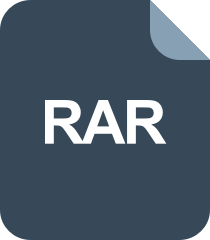
springboot集成mysql
在 Spring Boot 项目中,通常会采用 MyBatis 框架进行持久化操作,按照 MVC 架构的设计思路,可将代码按照功能分为 Entity、Mapper 和 Service 三个层次。
1. Entity
Entity 层是与数据库表进行映射的实体类。在 Entity 层中,通常会定义以下内容:
- 定义实体类的属性和对应的数据库表字段
- 定义实体类之间的关系
Entity 层的示例代码如下:
```
public class User {
private Long id;
private String name;
private Integer age;
// 省略 getter 和 setter 方法
}
```
2. Mapper
Mapper 层是连接数据库和业务逻辑的桥梁,它使用 MyBatis 框架来实现数据库操作。在 Mapper 层中,通常会定义以下内容:
- 定义 SQL 语句和参数映射
- 定义查询条件和排序方式
- 定义对数据库的增删改查操作
Mapper 层的示例代码如下:
```
@Mapper
public interface UserMapper {
User getUserById(Long id);
int addUser(User user);
int updateUser(User user);
int deleteUser(Long id);
}
```
3. Service
Service 层是业务逻辑的处理层,它主要负责处理业务逻辑和调用 Mapper 层完成数据库操作。在 Service 层中,通常会定义以下内容:
- 定义业务逻辑的方法
- 定义事务的管理方法
Service 层的示例代码如下:
```
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public User getUserById(Long id) {
return userMapper.getUserById(id);
}
public int addUser(User user) {
return userMapper.addUser(user);
}
public int updateUser(User user) {
return userMapper.updateUser(user);
}
public int deleteUser(Long id) {
return userMapper.deleteUser(id);
}
}
```
以上就是 Spring Boot 中 Entity、Mapper 和 Service 三个层次的概述和示例代码。需要注意的是,为了让 MyBatis 自动扫描 Mapper 接口并实例化,需要在启动类上添加 @MapperScan 注解,如下所示:
```
@SpringBootApplication
@MapperScan("com.example.demo.mapper")
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
其中,com.example.demo.mapper 是 Mapper 所在的包路径。
阅读全文
相关推荐
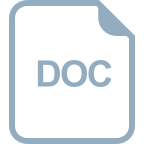
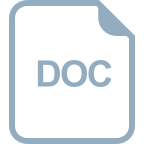

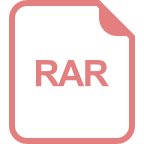
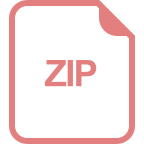
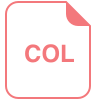








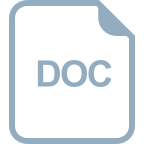
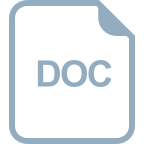
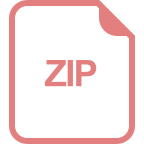