用两个栈来实现一个队列,使用n个元素来完成 n 次在队列尾部插入整数(push)和n次在队列头部删除整数(pop)的功能。 队列中的元素为int类型。保证操作合法,即保证pop操作时队列内已有元素。 数据范围: n≤1000
时间: 2024-05-02 13:20:46 浏览: 44
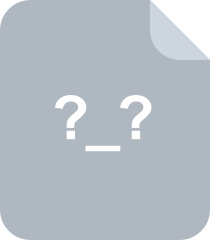
用两个栈实现一个队列的功能
可以使用两个栈stack1和stack2。当插入操作时,将元素压入stack1中。当删除操作时,如果stack2不为空,则弹出栈顶元素;如果stack2为空,则将stack1中的元素逐个弹出并压入stack2中,再弹出stack2的栈顶元素。
代码示例:
```cpp
#include<stack>
using namespace std;
class MyQueue {
public:
stack<int> stack1, stack2;
/** Initialize your data structure here. */
MyQueue() {
}
/** Push element x to the back of queue. */
void push(int x) {
stack1.push(x);
}
/** Removes the element from in front of queue and returns that element. */
int pop() {
if(stack2.empty()){
while(!stack1.empty()){
stack2.push(stack1.top());
stack1.pop();
}
}
int res=stack2.top();
stack2.pop();
return res;
}
/** Get the front element. */
int peek() {
int res=this->pop();
stack2.push(res);
return res;
}
/** Returns whether the queue is empty. */
bool empty() {
return stack1.empty()&&stack2.empty();
}
};
```
阅读全文
相关推荐





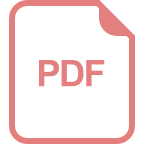
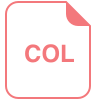







