Android 测试手机与指定MAC地址的智能血压计进行BLE蓝牙扫描、连接、认证、断开的代码
时间: 2023-07-10 10:09:34 浏览: 57
以下是一个基本的 Android BLE 蓝牙连接示例代码,用于连接指定 MAC 地址的智能血压计设备。请注意,此代码仅作为参考,具体实现可能因设备和 Android 版本而异。
首先,确保你的应用程序已经请求了蓝牙权限。
```
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
```
然后,在您的代码中,您需要使用 BluetoothAdapter 来执行扫描操作。在扫描期间,当您发现匹配的设备时,您可以使用 BluetoothDevice 对象连接到它。
```
private BluetoothAdapter mBluetoothAdapter;
private BluetoothGatt mBluetoothGatt;
private BluetoothDevice mDevice;
private String mDeviceAddress = "00:11:22:33:44:55";
// 初始化蓝牙适配器
final BluetoothManager bluetoothManager = (BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE);
mBluetoothAdapter = bluetoothManager.getAdapter();
// 开始扫描设备
mBluetoothAdapter.startLeScan(mLeScanCallback);
// 扫描回调
private BluetoothAdapter.LeScanCallback mLeScanCallback = new BluetoothAdapter.LeScanCallback() {
@Override
public void onLeScan(final BluetoothDevice device, int rssi, byte[] scanRecord) {
// 扫描到指定设备
if (device.getAddress().equals(mDeviceAddress)) {
mDevice = device;
// 停止扫描
mBluetoothAdapter.stopLeScan(mLeScanCallback);
// 连接设备
mBluetoothGatt = mDevice.connectGatt(getApplicationContext(), false, mGattCallback);
}
}
};
```
在连接到设备后,您需要使用 BluetoothGattCallback 来处理连接事件、服务发现和特征写入等操作。
```
private BluetoothGattCallback mGattCallback = new BluetoothGattCallback() {
// 连接状态改变
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
if (newState == BluetoothProfile.STATE_CONNECTED) {
// 连接成功,开始服务发现
gatt.discoverServices();
} else if (newState == BluetoothProfile.STATE_DISCONNECTED) {
// 连接断开
mBluetoothGatt.close();
mBluetoothGatt = null;
}
}
// 服务发现完成
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
if (status == BluetoothGatt.GATT_SUCCESS) {
// 在这里可以连接设备的服务和特征,然后进行读/写操作
BluetoothGattService service = mBluetoothGatt.getService(UUID.fromString(SERVICE_UUID));
BluetoothGattCharacteristic characteristic = service.getCharacteristic(UUID.fromString(CHARACTERISTIC_UUID));
gatt.setCharacteristicNotification(characteristic, true);
// 写入数据到设备
byte[] data = {0x01, 0x02, 0x03};
characteristic.setValue(data);
gatt.writeCharacteristic(characteristic);
}
}
// 特征写入完成
@Override
public void onCharacteristicWrite(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
// 写入完成后,可以断开连接
gatt.disconnect();
}
};
```
最后,如果需要,在断开连接时关闭 BluetoothGatt 对象。
```
if (mBluetoothGatt != null) {
mBluetoothGatt.close();
mBluetoothGatt = null;
}
```
相关推荐
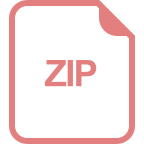
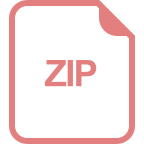
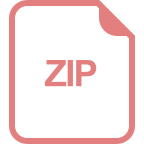














