在下边的代码中 如何释放myPicControl1.GetBitmap Bitmap myBmp = new Bitmap(filepath+ filetype_jpg); using (Graphics graphics = Graphics.FromImage(myBmp)) { Font font = new Font("Black", 80, FontStyle.Bold); System.Drawing.Brush brush = new SolidBrush(Color.FromArgb(128, Color.LightGray)); graphics.TranslateTransform(myBmp.Width / 2, myBmp.Height / 2); graphics.RotateTransform(330f); graphics.DrawString(watermark, font, brush, -2100, -100); //graphics.RotateTransform(135f); TabPage_JPG.Show(); } myPicControl1.GetBitmap = myBmp;
时间: 2023-12-10 11:03:16 浏览: 30
你可以在代码的结尾使用以下语句释放Bitmap对象:
```
myBmp.Dispose();
```
这将释放Bitmap对象占用的内存。当然,如果你使用的是using语句,程序会自动释放Bitmap对象,这样你就不需要手动释放它了。
```
using (Bitmap myBmp = new Bitmap(filepath+ filetype_jpg))
{
using (Graphics graphics = Graphics.FromImage(myBmp))
{
Font font = new Font("Black", 80, FontStyle.Bold);
System.Drawing.Brush brush = new SolidBrush(Color.FromArgb(128, Color.LightGray));
graphics.TranslateTransform(myBmp.Width / 2, myBmp.Height / 2);
graphics.RotateTransform(330f);
graphics.DrawString(watermark, font, brush, -2100, -100);
myPicControl1.GetBitmap = myBmp;
}
}
```
这样你就不需要手动释放Bitmap对象了。
相关问题
try { bitmap = MediaStore.Images.Media.getBitmap(getActivity().getContentResolver(), uri); // 将图片显示在 ImageView 控件中 imageView.setImageBitmap(bitmap); } catch (IOException e) { e.printStackTrace(); }
这段代码是从 MediaStore 中获取图片的 Bitmap 对象,并将其显示在 ImageView 控件中。具体地,它使用 getActivity().getContentResolver() 来获取 ContentResolver 对象,然后使用该对象和图片的 URI(Uniform Resource Identifier)来获取图片的 Bitmap 对象。如果获取成功,则将其设置为 ImageView 的图片。如果发生 IOException 异常,则打印堆栈跟踪并继续执行。
使用上面的代码后报错com.makeramen.roundedimageview.RoundedDrawable cannot be cast to android.graphics.drawable.BitmapDrawable
很抱歉,我的回答有误。如果你要从 RoundedImageView 中获取 Bitmap,你需要先将 RoundedDrawable 转换为 Bitmap,然后再获取 Bitmap。可以使用以下代码:
```java
RoundedImageView imageView = findViewById(R.id.image_view);
Drawable drawable = imageView.getDrawable();
Bitmap bitmap = null;
if (drawable instanceof RoundedDrawable) {
bitmap = ((RoundedDrawable) drawable).getSourceBitmap();
} else if (drawable instanceof BitmapDrawable) {
bitmap = ((BitmapDrawable) drawable).getBitmap();
}
```
这段代码首先检查 RoundedImageView 中的 Drawable 是否是 RoundedDrawable,如果是,就通过 getSourceBitmap() 方法获取 Bitmap。如果不是 RoundedDrawable,那么就检查 Drawable 是否是 BitmapDrawable,如果是,就通过 getBitmap() 方法获取 Bitmap。如果 Drawable 既不是 RoundedDrawable 也不是 BitmapDrawable,那么将 bitmap 设置为 null。
希望这次回答能够解决你的问题。
相关推荐
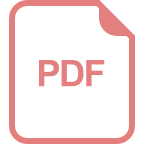
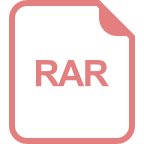
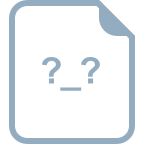












