用代码制作一个外围带有360°刻度线的圆,在圆心处有1个90°的扇形框,分别标识为G1。在圆上有1个随机的黑点,此系统拥有翻转按钮,若G1可以覆盖黑点,就将其移动到黑点处,并将G1变成红色.
时间: 2024-04-30 14:22:23 浏览: 76
以下是Python的实现代码:
``` python
import turtle
import random
# 绘制圆
def draw_circle():
turtle.penup()
turtle.goto(0, -200)
turtle.pendown()
turtle.circle(200)
# 绘制刻度线
def draw_scale():
turtle.penup()
turtle.goto(0, 0)
turtle.pendown()
for i in range(360):
turtle.forward(200)
turtle.backward(200)
turtle.right(1)
# 绘制G1
def draw_g1():
turtle.penup()
turtle.goto(0, 0)
turtle.pendown()
turtle.fillcolor("white")
turtle.begin_fill()
turtle.circle(100, 90)
turtle.right(90)
turtle.forward(100)
turtle.left(90)
turtle.end_fill()
turtle.penup()
turtle.goto(0, 0)
turtle.pendown()
turtle.right(90)
# 绘制黑点
def draw_black_dot():
turtle.penup()
turtle.goto(0, 0)
turtle.pendown()
turtle.dot(10, "black")
# 移动G1到黑点
def move_g1_to_black_dot():
turtle.penup()
turtle.goto(0, 0)
turtle.pendown()
turtle.fillcolor("red")
turtle.begin_fill()
turtle.circle(100, 90)
turtle.right(90)
turtle.forward(100)
turtle.left(90)
turtle.end_fill()
turtle.penup()
turtle.goto(black_dot_position)
turtle.pendown()
turtle.fillcolor("red")
turtle.begin_fill()
turtle.circle(10)
turtle.end_fill()
# 系统翻转按钮
def flip():
global g1_color
if g1_color == "white":
if check_overlap():
move_g1_to_black_dot()
g1_color = "red"
else:
draw_g1()
g1_color = "white"
# 检查G1是否覆盖黑点
def check_overlap():
if turtle.distance(black_dot_position) < 100 and 0 < turtle.towards(black_dot_position) < 90:
return True
else:
return False
# 初始化
turtle.speed(0)
draw_circle()
draw_scale()
draw_g1()
# 随机生成黑点位置
black_dot_position = (random.randint(-180, 180), random.randint(-180, 180))
draw_black_dot()
# 系统初始状态
g1_color = "white"
# 监听翻转按钮
turtle.onkey(flip, "space")
turtle.listen()
turtle.mainloop()
```
代码说明:
首先,我们引入了turtle模块,turtle是Python自带的一个绘图模块,可以用来绘制各种图形。
然后,我们定义了几个函数,分别用于绘制圆、刻度线、G1、黑点、移动G1到黑点等操作。其中,check_overlap函数用于检查G1是否覆盖黑点,flip函数用于实现系统的翻转按钮。
在主程序中,我们先绘制了圆、刻度线和G1,然后随机生成黑点的位置并绘制出来。系统初始状态下,G1是白色的,表示没有覆盖黑点。然后,我们监听空格键,当用户按下空格键时,系统执行翻转操作,如果G1覆盖了黑点,就将G1移动到黑点处并将其变成红色,否则就重新绘制G1并将其变成白色。
最后,我们启动turtle的主循环,让程序一直运行,直到用户关闭窗口。
阅读全文
相关推荐
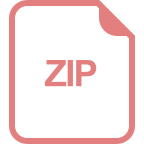








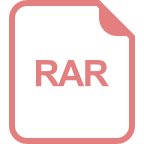
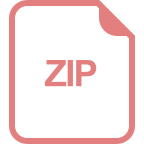
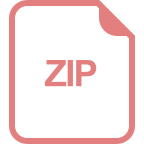
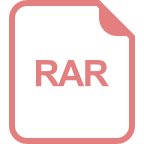
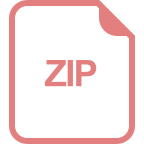
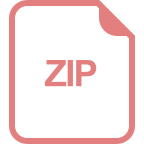


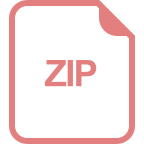