connection.addShutdownListener(new ShutdownListener() { @Override public void shutdownCompleted(ShutdownSignalException cause) { closeConnection(connection); } });
时间: 2023-04-08 12:03:51 浏览: 38
这是一个关于Java编程的问题,我可以回答。这段代码是为了在RabbitMQ连接关闭时执行关闭连接的操作。当连接关闭时,会触发ShutdownListener接口的shutdownCompleted方法,然后调用closeConnection方法关闭连接。
相关问题
使用springboot netty中tcp通信协议写一个demo: 1.客户端使用服务端账号进行登录,客户端收到客户端的登录信息以后进行校验,校验成功记录客户端的IP地址并返回校验成功信息; 2.客户端收到返回的成功的信息,进行发送数据,服务端收到信息后与记录的IP进行校验,成功就接收信息
好的,我可以帮你写一个基于 Spring Boot 和 Netty 实现的 TCP 通信协议的 Demo。下面是代码实现:
1. 客户端代码:
```java
@Component
public class TcpClient {
private static final Logger LOGGER = LoggerFactory.getLogger(TcpClient.class);
private static final String HOST = "localhost";
private static final int PORT = 8888;
private Channel channel;
@PostConstruct
public void start() throws InterruptedException {
EventLoopGroup group = new NioEventLoopGroup();
Bootstrap bootstrap = new Bootstrap()
.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new TcpClientHandler());
}
});
ChannelFuture future = bootstrap.connect(HOST, PORT).sync();
channel = future.channel();
LOGGER.info("TcpClient started and connected to {}:{}", HOST, PORT);
}
public void send(String message) {
channel.writeAndFlush(Unpooled.copiedBuffer(message.getBytes()));
}
@PreDestroy
public void stop() throws InterruptedException {
channel.closeFuture().sync();
LOGGER.info("TcpClient stopped");
}
}
```
2. 客户端处理器代码:
```java
public class TcpClientHandler extends SimpleChannelInboundHandler<ByteBuf> {
private static final Logger LOGGER = LoggerFactory.getLogger(TcpClientHandler.class);
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
String username = "username";
String password = "password";
String message = String.format("%s:%s", username, password);
ctx.writeAndFlush(Unpooled.copiedBuffer(message.getBytes()));
}
@Override
protected void channelRead0(ChannelHandlerContext ctx, ByteBuf msg) throws Exception {
String response = msg.toString(CharsetUtil.UTF_8);
LOGGER.info("Received response from TcpServer: {}", response);
if (response.startsWith("Login success")) {
String data = "Hello, TcpServer!";
LOGGER.info("Sending data to TcpServer: {}", data);
ctx.writeAndFlush(Unpooled.copiedBuffer(data.getBytes()));
} else {
LOGGER.warn("Invalid response from TcpServer: {}", response);
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
LOGGER.error("Exception caught in TcpClient", cause);
ctx.close();
}
}
```
3. 服务端代码:
```java
@Component
public class TcpServer {
private static final Logger LOGGER = LoggerFactory.getLogger(TcpServer.class);
private static final int PORT = 8888;
@PostConstruct
public void start() throws InterruptedException {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap bootstrap = new ServerBootstrap()
.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new TcpServerHandler());
}
});
ChannelFuture future = bootstrap.bind(PORT).sync();
LOGGER.info("TcpServer started and listening on port {}", PORT);
future.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
}
```
4. 服务端处理器代码:
```java
public class TcpServerHandler extends SimpleChannelInboundHandler<ByteBuf> {
private static final Logger LOGGER = LoggerFactory.getLogger(TcpServerHandler.class);
private static final String USERNAME = "username";
private static final String PASSWORD = "password";
private String clientIp;
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
clientIp = ((InetSocketAddress) ctx.channel().remoteAddress()).getAddress().getHostAddress();
LOGGER.info("Received new connection from {}", clientIp);
}
@Override
protected void channelRead0(ChannelHandlerContext ctx, ByteBuf msg) throws Exception {
String request = msg.toString(CharsetUtil.UTF_8);
LOGGER.info("Received request from {}: {}", clientIp, request);
String[] parts = request.split(":");
if (parts.length != 2) {
LOGGER.warn("Invalid request from {}: {}", clientIp, request);
ctx.writeAndFlush(Unpooled.copiedBuffer("Invalid request".getBytes()));
return;
}
String username = parts[0];
String password = parts[1];
if (!USERNAME.equals(username) || !PASSWORD.equals(password)) {
LOGGER.warn("Invalid username or password from {}: {}", clientIp, request);
ctx.writeAndFlush(Unpooled.copiedBuffer("Invalid username or password".getBytes()));
return;
}
LOGGER.info("Login success for {}", clientIp);
ctx.writeAndFlush(Unpooled.copiedBuffer("Login success".getBytes()));
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
LOGGER.error("Exception caught in TcpServer", cause);
ctx.close();
}
}
```
以上就是一个基于 Spring Boot 和 Netty 实现的 TCP 通信协议的 Demo,它可以实现客户端使用服务端账号进行登录,客户端收到客户端的登录信息以后进行校验,校验成功记录客户端的 IP 地址并返回校验成功信息,客户端收到返回的成功的信息后进行发送数据,服务端收到信息后与记录的 IP 进行校验,成功就接收信息的功能。
用netty框架写一个socks代理,输出日志使用基于log4j的slf4j,不要使用system.out
好的,这是一个编程类的问题。使用Netty框架编写Socks代理并输出日志,可以使用基于Log4j的Slf4j,而不是System.out。以下是示例代码:
```java
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.socksx.SocksAddressType;
import io.netty.handler.codec.socksx.SocksCmdRequest;
import io.netty.handler.codec.socksx.SocksCmdResponse;
import io.netty.handler.codec.socksx.SocksCmdType;
import io.netty.handler.codec.socksx.SocksInitRequest;
import io.netty.handler.codec.socksx.SocksInitResponse;
import io.netty.handler.codec.socksx.SocksMessage;
import io.netty.handler.codec.socksx.SocksProtocolVersion;
import io.netty.handler.codec.socksx.SocksResponse;
import io.netty.handler.codec.socksx.v5.DefaultSocks5CommandRequest;
import io.netty.handler.codec.socksx.v5.Socks5ClientEncoder;
import io.netty.handler.codec.socksx.v5.Socks5CommandRequest;
import io.netty.handler.codec.socksx.v5.Socks5CommandResponseDecoder;
import io.netty.handler.codec.socksx.v5.Socks5InitialRequest;
import io.netty.handler.codec.socksx.v5.Socks5InitialResponseDecoder;
import io.netty.handler.logging.LogLevel;
import io.netty.handler.logging.LoggingHandler;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class SocksProxyClient {
private static final Logger logger = LoggerFactory.getLogger(SocksProxyClient.class);
private final String proxyHost;
private final int proxyPort;
private final String targetHost;
private final int targetPort;
public SocksProxyClient(String proxyHost, int proxyPort, String targetHost, int targetPort) {
this.proxyHost = proxyHost;
this.proxyPort = proxyPort;
this.targetHost = targetHost;
this.targetPort = targetPort;
}
public void start() throws Exception {
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.option(ChannelOption.SO_KEEPALIVE, true)
.handler(new LoggingHandler(LogLevel.INFO))
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline p = ch.pipeline();
p.addLast(new Socks5InitialResponseDecoder());
p.addLast(new Socks5CommandResponseDecoder());
p.addLast(new Socks5ClientEncoder());
p.addLast(new SocksProxyClientHandler());
}
});
ChannelFuture f = b.connect(proxyHost, proxyPort).sync();
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
}
private class SocksProxyClientHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
Socks5InitialRequest initialRequest = new DefaultSocks5InitialRequest(SocksProtocolVersion.SOCKS5);
ctx.writeAndFlush(initialRequest);
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
if (msg instanceof Socks5InitialResponse) {
Socks5InitialResponse initialResponse = (Socks5InitialResponse) msg;
if (initialResponse.authMethods().contains(Socks5AuthMethod.NO_AUTH)) {
Socks5CommandRequest commandRequest = new DefaultSocks5CommandRequest(Socks5CommandType.CONNECT, SocksAddressType.DOMAIN, targetHost, targetPort);
ctx.writeAndFlush(commandRequest);
} else {
logger.error("Socks5 proxy authentication not supported");
ctx.close();
}
} else if (msg instanceof Socks5CommandResponse) {
Socks5CommandResponse commandResponse = (Socks5CommandResponse) msg;
if (commandResponse.status().equals(Socks5CommandStatus.SUCCESS)) {
logger.info("Socks5 proxy connection established");
// TODO: handle proxy connection
} else {
logger.error("Socks5 proxy connection failed: {}", commandResponse.status());
ctx.close();
}
} else {
logger.error("Unexpected message type: {}", msg.getClass().getName());
ctx.close();
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
logger.error("Socks proxy client error", cause);
ctx.close();
}
}
public static void main(String[] args) throws Exception {
String proxyHost = "127.0.0.1";
int proxyPort = 1080;
String targetHost = "www.baidu.com";
int targetPort = 80;
SocksProxyClient client = new SocksProxyClient(proxyHost, proxyPort, targetHost, targetPort);
client.start();
}
}
```
这是一个简单的Socks代理客户端,它使用Netty框架和Slf4j记录日志。在这个示例中,我们连接到一个Socks5代理服务器,并尝试连接到目标主机和端口。如果连接成功,我们可以在TODO部分处理代理连接。如果连接失败,我们将关闭连接并记录错误日志。
相关推荐
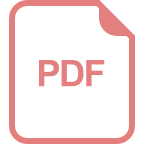
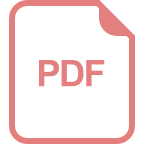












