python 第三次大作业:用按键,做一个排雷的程序,棋盘大小10*10的,随机生成25个雷,然后排雷,要有左键点开,右键标记的功能
时间: 2023-08-22 09:05:52 浏览: 41
好的,以下是完整的 Python 代码。你可以将其复制到 Python 编辑器中,保存为 .py 文件,然后运行它来体验排雷游戏。
```python
import tkinter as tk
import random
# 创建窗口
root = tk.Tk()
root.title("Minesweeper")
root.geometry("500x500")
# 创建标题
title_label = tk.Label(root, text="Minesweeper", font=("Arial", 24))
title_label.pack()
# 创建棋盘
board = []
for i in range(10):
row = []
for j in range(10):
button = tk.Button(root, text="", width=3, height=2)
button.grid(row=i+1, column=j+1)
button.bind("<Button-1>", left_click)
button.bind("<Button-3>", right_click)
row.append(button)
board.append(row)
# 随机生成 25 个雷
mines = set()
while len(mines) < 25:
i = random.randint(0, 9)
j = random.randint(0, 9)
if (i, j) not in mines:
mines.add((i, j))
# 左键点击事件
def left_click(event):
button = event.widget
i, j = get_index(button)
if (i, j) in mines:
button.config(text="X", bg="red")
game_over()
else:
count = count_mines(i, j)
button.config(text=count, bg="white")
# 右键点击事件
def right_click(event):
button = event.widget
i, j = get_index(button)
if button.cget("text") == "":
button.config(text="*", fg="red")
elif button.cget("text") == "*":
button.config(text="?", fg="blue")
else:
button.config(text="", fg="black")
# 计算周围的雷数
def count_mines(i, j):
count = 0
for a, b in [(i-1, j-1), (i-1, j), (i-1, j+1), (i, j-1), (i, j+1), (i+1, j-1), (i+1, j), (i+1, j+1)]:
if 0 <= a < 10 and 0 <= b < 10 and (a, b) in mines:
count += 1
return count
# 游戏结束
def game_over():
for i in range(10):
for j in range(10):
if (i, j) in mines:
board[i][j].config(text="*", bg="white")
else:
count = count_mines(i, j)
board[i][j].config(text=count, bg="white")
# 获取按钮在二维数组中的索引
def get_index(button):
for i in range(10):
for j in range(10):
if board[i][j] == button:
return i, j
# 启动游戏
root.mainloop()
```
希望这个排雷游戏能够满足你的需求,有什么问题可以随时提出来。
相关推荐
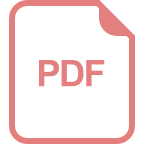










