用C语言写一个中值滤波程序
时间: 2023-09-10 15:13:35 浏览: 179
以下是使用 C 语言实现的简单中值滤波程序:
```c
#include <stdio.h>
#include <stdlib.h>
#define KERNEL_SIZE 3
int compare(const void *a, const void *b)
{
return (*(int*)a - *(int*)b);
}
void median_filter(unsigned char *input, int width, int height, unsigned char *output)
{
int i, j, k, l, p, q;
int padding_size = KERNEL_SIZE / 2;
unsigned char roi[KERNEL_SIZE * KERNEL_SIZE];
for (i = padding_size; i < height - padding_size; i++) {
for (j = padding_size; j < width - padding_size; j++) {
p = 0;
for (k = -padding_size; k <= padding_size; k++) {
for (l = -padding_size; l <= padding_size; l++) {
roi[p++] = input[(i + k) * width + (j + l)];
}
}
qsort(roi, KERNEL_SIZE * KERNEL_SIZE, sizeof(unsigned char), compare);
output[i * width + j] = roi[KERNEL_SIZE * KERNEL_SIZE / 2];
}
}
}
int main()
{
FILE *fp_in, *fp_out;
unsigned char *input, *output;
int width, height;
int i, j;
fp_in = fopen("lena.raw", "rb");
if (fp_in == NULL) {
printf("Error: Unable to open input file.\n");
return 1;
}
fp_out = fopen("lena_filtered.raw", "wb");
if (fp_out == NULL) {
printf("Error: Unable to open output file.\n");
fclose(fp_in);
return 1;
}
width = height = 512;
input = (unsigned char*)malloc(width * height * sizeof(unsigned char));
output = (unsigned char*)malloc(width * height * sizeof(unsigned char));
fread(input, sizeof(unsigned char), width * height, fp_in);
median_filter(input, width, height, output);
fwrite(output, sizeof(unsigned char), width * height, fp_out);
free(input);
free(output);
fclose(fp_in);
fclose(fp_out);
return 0;
}
```
该程序从文件读取输入图像,对其进行中值滤波,并将处理结果写入输出文件。中值滤波的核心函数 `median_filter` 接受四个参数:输入图像、图像宽度、图像高度和输出图像。在函数内部,我们遍历每个像素,并将滤波器中的像素进行排序,取中间值作为该像素的新值。排序使用了标准库中的 `qsort` 函数。
请注意,由于中值滤波涉及到像素排序操作,因此在实际应用中,为了提高程序效率,通常会使用一些优化技术,例如快速中值滤波(FMF)等。
阅读全文
相关推荐
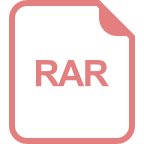
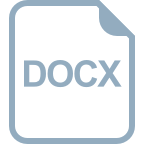
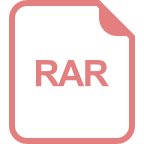
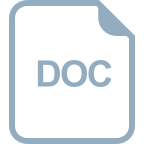


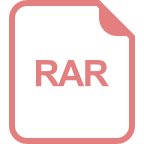
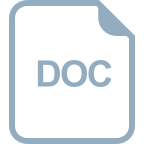
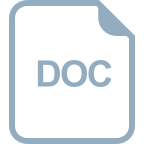
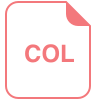





