帮我写一个U_Net网络训练程序
时间: 2024-03-27 17:40:27 浏览: 17
好的,这里提供一个简单的U-Net网络训练程序,使用PyTorch框架编写。
首先需要导入必要的库:
```python
import torch
import torch.nn as nn
import torch.optim as optim
from torch.utils.data import DataLoader
from torchvision import transforms
from torchvision.datasets import ImageFolder
```
接下来定义U-Net网络的结构,包括下采样模块、上采样模块和跳跃连接:
```python
class UNet(nn.Module):
def __init__(self):
super(UNet, self).__init__()
self.down1 = Down(3, 64)
self.down2 = Down(64, 128)
self.down3 = Down(128, 256)
self.down4 = Down(256, 512)
self.bottom = Bottom(512, 1024)
self.up1 = Up(1024, 512)
self.up2 = Up(512, 256)
self.up3 = Up(256, 128)
self.up4 = Up(128, 64)
self.output = nn.Conv2d(64, 1, kernel_size=1, stride=1, padding=0)
def forward(self, x):
x1 = self.down1(x)
x2 = self.down2(x1)
x3 = self.down3(x2)
x4 = self.down4(x3)
x5 = self.bottom(x4)
x6 = self.up1(x5, x4)
x7 = self.up2(x6, x3)
x8 = self.up3(x7, x2)
x9 = self.up4(x8, x1)
out = self.output(x9)
return out
```
下采样模块使用两个卷积层加一个最大池化层,上采样模块使用一个反卷积层加一个卷积层,跳跃连接将下采样模块的输出与上采样模块的输入相加。
```python
class Down(nn.Module):
def __init__(self, in_channels, out_channels):
super(Down, self).__init__()
self.conv1 = nn.Conv2d(in_channels, out_channels, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(out_channels, out_channels, kernel_size=3, stride=1, padding=1)
self.pool = nn.MaxPool2d(kernel_size=2, stride=2)
def forward(self, x):
x = self.conv1(x)
x = nn.ReLU(inplace=True)(x)
x = self.conv2(x)
x = nn.ReLU(inplace=True)(x)
down = self.pool(x)
return down, x
class Bottom(nn.Module):
def __init__(self, in_channels, out_channels):
super(Bottom, self).__init__()
self.conv1 = nn.Conv2d(in_channels, out_channels, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(out_channels, out_channels, kernel_size=3, stride=1, padding=1)
def forward(self, x):
x = self.conv1(x)
x = nn.ReLU(inplace=True)(x)
x = self.conv2(x)
x = nn.ReLU(inplace=True)(x)
return x
class Up(nn.Module):
def __init__(self, in_channels, out_channels):
super(Up, self).__init__()
self.up = nn.ConvTranspose2d(in_channels, out_channels, kernel_size=2, stride=2)
self.conv1 = nn.Conv2d(in_channels, out_channels, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(out_channels, out_channels, kernel_size=3, stride=1, padding=1)
def forward(self, x, skip):
x = self.up(x)
x = torch.cat([x, skip], dim=1)
x = self.conv1(x)
x = nn.ReLU(inplace=True)(x)
x = self.conv2(x)
x = nn.ReLU(inplace=True)(x)
return x
```
接下来定义数据集和数据预处理:
```python
train_transforms = transforms.Compose([
transforms.Resize((256, 256)),
transforms.RandomHorizontalFlip(),
transforms.ToTensor()
])
test_transforms = transforms.Compose([
transforms.Resize((256, 256)),
transforms.ToTensor()
])
train_dataset = ImageFolder(root='train/', transform=train_transforms)
test_dataset = ImageFolder(root='test/', transform=test_transforms)
train_dataloader = DataLoader(train_dataset, batch_size=16, shuffle=True)
test_dataloader = DataLoader(test_dataset, batch_size=16, shuffle=False)
```
定义损失函数和优化器:
```python
model = UNet().cuda()
criterion = nn.BCEWithLogitsLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
```
训练模型:
```python
for epoch in range(10):
running_loss = 0.0
for i, data in enumerate(train_dataloader, 0):
inputs, labels = data
inputs, labels = inputs.cuda(), labels.cuda()
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels.float().unsqueeze(1))
loss.backward()
optimizer.step()
running_loss += loss.item()
print('Epoch %d loss: %.3f' % (epoch + 1, running_loss / len(train_dataloader)))
print('Finished Training')
```
以上是一个简单的U-Net网络训练程序,可以根据具体任务和数据集进行调整。
相关推荐
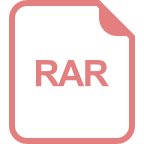
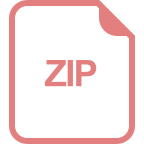














