分析下列代码:#include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> #include <netdb.h> #define SERVER_PORT 12345 /* arbitrary, but client and server must agree */ #define BUF_SIZE 4096 /* block transfer size */ int main(int argc, char **argv) { int c, s, bytes; char buf[BUF_SIZE]; /* buffer for incoming file */ struct hostent *h; /* info about server */ struct sockaddr_in channel; /* holds IP address */ if (argc != 3) fatal("Usage: client server-name file-name"); h = gethostbyname(argv[1]); /* look up host's IP address */ if (!h) fatal("gethostbyname failed"); s = socket(PF_INET, SOCK_STREAM, IPPROTO_TCP); if (s < 0) fatal("socket"); memset(&channel, 0, sizeof(channel)); channel.sin_family= AF_INET; memcpy(&channel.sin_addr.s_addr, h->h_addr, h->h_length); channel.sin_port= htons(SERVER_PORT); c = connect(s, (struct sockaddr *) &channel, sizeof(channel)); if (c < 0) fatal("connect failed"); /* Connection is now established. Send file name including 0 byte at end. */ write(s, argv[2], strlen(argv[2])+1); /* Go get the file and write it to standard output. */ while (1) { bytes = read(s, buf, BUF_SIZE); /* read from socket */ if (bytes <= 0) exit(0); /* check for end of file */ write(1, buf, bytes); /* write to standard output */ } } fatal(char *string) { printf("%s\n", string); exit(1); }
时间: 2024-04-01 22:36:53 浏览: 62
这段代码实现了一个基于TCP协议的客户端,用于从服务器上获取文件并将文件输出到标准输出。具体实现过程如下:
1. 包含了必要的头文件。
2. 定义了服务器端口号和缓冲区大小常量。
3. 实现了一个fatal函数,用于在出现错误时输出错误信息并退出程序。
4. 在main函数中,首先根据命令行参数获取服务器的IP地址,并检查是否正确获取。
5. 创建一个TCP套接字,连接到服务器上。
6. 将文件名通过套接字发送到服务器端。
7. 在一个循环中,从套接字中读取数据,并将读取到的数据输出到标准输出中,直到读取到文件末尾。
需要注意的是,该代码没有进行错误处理,例如在读取文件时可能会出现错误。因此,在实际应用中需要增加相应的错误处理代码。
相关问题
解释以下头文件:#include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> #include <unistd.h> #include <fcntl.h> #include <pthread.h> #include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> #include <ctype.h> #include <sys/pps.h> #include <sys/types.h> #include "camera.h" #include <encoder.h> #include "ppsFunc.h" #include "timeDiff.h"
这些头文件是C语言程序中常用的系统库和自定义头文件。下面对它们进行简要的解释:
- stdio.h:输入输出相关的函数库,如printf、scanf等。
- stdlib.h:标准库函数,包括各种常用函数,如malloc、free等。
- stdint.h:定义了一些整数类型,如int8_t、uint16_t等。
- string.h:提供字符串操作相关的函数,如strcpy、strcat等。
- unistd.h:提供对POSIX操作系统API的访问,如close、read等。
- fcntl.h:提供对文件控制相关的函数,如open、fcntl等。
- pthread.h:多线程相关的函数库,如pthread_create、pthread_join等。
- sys/types.h:提供对系统数据类型的定义,如pid_t、mode_t等。
- sys/socket.h:提供对套接字的操作,如socket、bind等。
- netinet/in.h:提供对TCP/IP网络编程的支持,如struct sockaddr_in等。
- ctype.h:提供对字符分类和转换的函数,如isalpha、isdigit等。
- sys/pps.h:提供对PPS(Pulse-Per-Second)信号的读取和控制。
- camera.h:自定义头文件,可能是和摄像头相关的函数和数据结构。
- encoder.h:自定义头文件,可能是和视频编码相关的函数和数据结构。
- ppsFunc.h:自定义头文件,可能是和PPS信号相关的函数和数据结构。
- timeDiff.h:自定义头文件,可能是和时间差相关的函数和数据结构。
#include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> #include <unistd.h> #include <fcntl.h> #include <pthread.h> #include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> #include <ctype.h> #include <sys/pps.h> #include <sys/types.h> #include "camera.h" #include <encoder.h> #include "ppsFunc.h" #include "timeDiff.h"
这是一段C语言的代码,使用了一些系统库和自定义头文件。其中包括了网络编程相关的库,如sys/socket.h和netinet/in.h,以及多线程相关的库pthread.h。另外还有一些自定义的头文件,如camera.h和encoder.h,可能是该程序所需的一些外部依赖。该程序可能是一个视频流的采集和编码程序,同时还使用了PPS和时间差等功能。
阅读全文
相关推荐
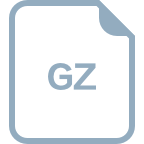
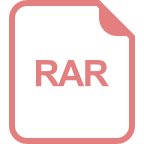
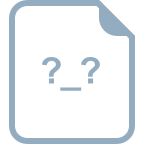













