color = np.stack(color, axis=0)
时间: 2024-01-27 21:06:18 浏览: 120
这行代码的作用是将一个列表中的多个 numpy 数组沿着新的轴合并成一个更高维度的数组。具体来说,axis=0 参数表示在新数组的第 0 个维度上堆叠原来的数组,也就是把原来的多个一维数组变成一个二维数组,其中每一行是原来的一个数组。
举个例子,如果 color 是一个包含 3 个一维数组的列表,每个数组都有 4 个元素,那么执行该代码后,color 变成了一个二维数组,其形状为 (3, 4),其中第一行对应原来列表中的第一个数组,第二行对应第二个数组,以此类推。
相关问题
import numpy as np import matplotlib.pyplot as plt import pywt from skimage import io, color # 读取灰度图像并转换为RGB图像 img_gray = io.imread('lena.png', as_gray=True) img = color.gray2rgb(img_gray) # 对图像的三个通道进行DWT变换 coeffs_r = pywt.dwt2(img[:, :, 0], 'haar') coeffs_g = pywt.dwt2(img[:, :, 1], 'haar') coeffs_b = pywt.dwt2(img[:, :, 2], 'haar') # 设置压缩比率 compress_ratio = 0.5 # 计算阈值 threshold_r = np.sort(np.abs(coeffs_r[1].ravel()))[::-1][int(compress_ratio * len(coeffs_r[1].ravel()))] threshold_g = np.sort(np.abs(coeffs_g[1].ravel()))[::-1][int(compress_ratio * len(coeffs_g[1].ravel()))] threshold_b = np.sort(np.abs(coeffs_b[1].ravel()))[::-1][int(compress_ratio * len(coeffs_b[1].ravel()))] # 对小于阈值的系数进行置零 coeffs_r = list(coeffs_r) coeffs_r[0] = np.round(coeffs_r[0]) coeffs_r[1] = np.where(np.abs(coeffs_r[1]) < threshold_r, 0, coeffs_r[1]) coeffs_r[2] = np.where(np.abs(coeffs_r[2]) < threshold_r, 0, coeffs_r[2]) coeffs_g = list(coeffs_g) coeffs_g[0] = np.round(coeffs_g[0]) coeffs_g[1] = np.where(np.abs(coeffs_g[1]) < threshold_g, 0, coeffs_g[1]) coeffs_g[2] = np.where(np.abs(coeffs_g[2]) < threshold_g, 0, coeffs_g[2]) coeffs_b = list(coeffs_b) coeffs_b[0] = np.round(coeffs_b[0]) coeffs_b[1] = np.where(np.abs(coeffs_b[1]) < threshold_b, 0, coeffs_b[1]) coeffs_b[2] = np.where(np.abs(coeffs_b[2]) < threshold_b, 0, coeffs_b[2]) # 合并三个通道的系数 coeffs = [np.stack([coeffs_r[i], coeffs_g[i], coeffs_b[i]], axis=-1) for i in range(len(coeffs_r))] # 对图像进行IDWT反变换 img_dwt = pywt.idwt2(coeffs, 'haar') # 显示原始图像和压缩后的图像 fig, axes = plt.subplots(nrows=1, ncols=2, figsize=(8, 4)) ax = axes.ravel() ax[0].imshow(img) ax[0].set_title("Original image") ax[1].imshow(img_dwt) ax[1].set_title("Compressed image (DWT)") ax[1].set_xlabel("Compression ratio: {:.2f}".format(compress_ratio)) plt.tight_layout() plt.show()
这段代码缺少了两行,分别是导入numpy和matplotlib.pyplot两个模块的语句。请在代码的开头添加以下两行语句:
```
import numpy as np
import matplotlib.pyplot as plt
```
这样就可以解决代码中的报错。
def drawPlot(title,x_train,x_test,y_train,y_test): N,M=500,500 x1_min,x2_min=x_train.min() x1_max,x2_max=x_train.max() t1=np.linspace(x1_min,x1_max,N) t2=np.linspace(x2_min,x2_max,M) x1,x2=np.meshgrid(t1,t2) x_show=np.stack((x1.flat,x2.flat),axis=1) y_hat=model.predict(x_show) y_hat=y_hat.reshape(x1.shape) cm_light=mpl.colors.ListedColormap(['#F2F2C2','#E00E00','#FFFFFF']) cm_dark=mpl.colors.ListedColormap(['#FFFFFF','#000000','#CCCCCC']) plt.figure(figsize=(3,2.5),facecolor='w') plt.contour(x1,x2,y_hat,colors='k',levels=[0,1],antialiased=True,linewidths=1) plt.pcolormesh(x1,x2,y_hat,cmap=cm_light) plt.scatter(x_train[0],x_train[1],c=y_train,s=20,edgecolors='k',cmap=cm_dark,label='训练集') plt.scatter(x_test[0],x_test[1],c=y_test,s=80,marker='*',edgecolors='k',cmap=cm_dark,label='测试集') plt.xlabel('花萼长度',fontsize=9) plt.ylabel('花萼宽度',fontsize=9) plt.xlim(x1_min,x1_max) plt.ylim(x2_min,x2_max) plt.grid(b=True,ls=':',color='#606060') plt.suptitle(title,fontsize=8) plt.tight_layout(1,rect=(0,0,1,0.94)) plt.show()报错TypeError: tight_layout() takes 0 positional arguments but 1 positional argument (and 1 keyword-only argument) were given
这个错误是因为 tight_layout() 这个函数需要传入一个子图布局的矩形参数,但是在这里没有给定。你可以将代码中的 plt.tight_layout(1,rect=(0,0,1,0.94)) 修改为 plt.tight_layout(rect=(0,0,1,0.94)),这样就可以解决这个问题了。
阅读全文
相关推荐
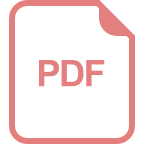
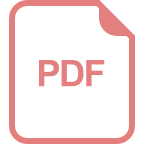
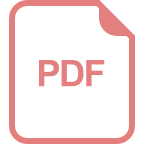

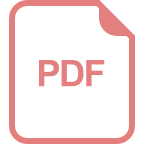
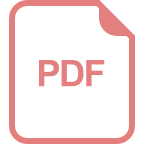







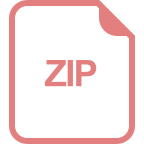
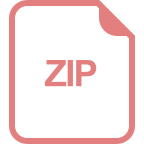
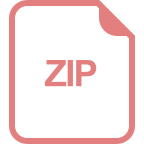
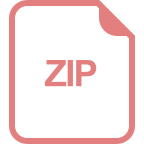