优化以下代码:#include <iostream> #include<string.h> using namespace std; class Book { private: char bookname[30]; char authers[30]; char publishing_house[40]; int pages; float price; public: char getbookname(); char getauthers(); char getpublishing_house(); int getpages(); float getprice(); void setbookname(char *a); void setauthers(char *a); void setpublishing_house(char *a); void setpages(int a); void setprice(float a); Book(char*a,char*b,cahr*c,int d,float e) { strcpy(bookname,a); strcpy(authers,b); strcpy(publishing_house,c); pages=d; price=e; } }; char getbookname() { char*a=bookname; return a; } char getauthers() { char*a=authers; return a; } char getpublishing_house() { char*a=publishing_house; return a; } int getpages() { int a=pages; return a; } float getprice() { float a=price; return a; } void setbookname(char *a) { strcpy(bookname,a); } void setauthers(char *a) { strcpy(authers,a); } void setpublishing_house(char *a) { strcpy(publishing_house,a); } void setpages(int a) { pages=a; } void setprice(float a) { price=a; } int main() { Book a(char a="《C++程序设计》",char b="谭浩强编制",char c="清华大学出版社",d=484,e=36.00); Book b(char a="《吹牛大王历险记》",char b="拉斯伯等编著",char c="天津人民出版社",d=149,e=12.80); cout<<a.getbookname()<<" "<<a.getauthers()<<" "<<a.getpublishing_house()<<" "<<"页数:"<<a.getpages()<<"价钱:"<<a.getprice()<<endl; cout<<b.getbookname()<<" "<<b.getauthers()<<" "<<b.getpublishing_house()<<" "<<"页数:"<<b.getpages()<<"价钱:"<<b.getprice()<<endl; return 0; }
时间: 2023-07-15 11:11:03 浏览: 83
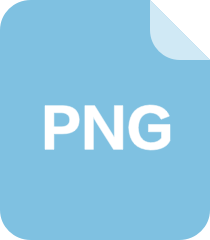
#include <iostream> #include<iomanip> using namespace std;九九乘法表
以下是优化后的代码:
#include<iostream>
#include<string.h>
using namespace std;
class Book {
private:
char bookname[30];
char authers[30];
char publishing_house[40];
int pages;
float price;
public:
Book(char* a="《C++程序设计》", char* b="谭浩强编制", char* c="清华大学出版社", int d=484, float e=36.00) {
strcpy(bookname, a);
strcpy(authers, b);
strcpy(publishing_house, c);
pages = d;
price = e;
}
char* getbookname() {
return bookname;
}
char* getauthers() {
return authers;
}
char* getpublishing_house() {
return publishing_house;
}
int getpages() {
return pages;
}
float getprice() {
return price;
}
void setbookname(char* a) {
strcpy(bookname, a);
}
void setauthers(char* a) {
strcpy(authers, a);
}
void setpublishing_house(char* a) {
strcpy(publishing_house, a);
}
void setpages(int a) {
pages = a;
}
void setprice(float a) {
price = a;
}
};
int main() {
Book a;
Book b("《吹牛大王历险记》", "拉斯伯等编著", "天津人民出版社", 149, 12.80);
cout << a.getbookname() << " " << a.getauthers() << " " << a.getpublishing_house() << " " << "页数:" << a.getpages() << "价钱:" << a.getprice() << endl;
cout << b.getbookname() << " " << b.getauthers() << " " << b.getpublishing_house() << " " << "页数:" << b.getpages() << "价钱:" << b.getprice() << endl;
return 0;
}
主要的修改如下:
1. 在类定义中,将所有的成员函数都定义在类的内部,并修改它们的访问修饰符为 public。
2. 在类定义中,将构造函数的参数都设置默认值,以免在创建对象的时候不必要地传递参数。
3. 在类定义中,将所有的成员变量都设置为 private。
4. 在类定义中,修改字符串类型成员变量的 getter 和 setter 函数,使它们返回和接收字符指针类型的数据。
5. 在类定义中,修改整型和浮点型成员变量的 getter 和 setter 函数,使它们返回和接收对应的数据类型。
6. 在主函数中,第一个 Book 对象 a 的构造函数调用中去掉了赋值语句,因为这些赋值已经在类的构造函数中完成了。
7. 在主函数中,第二个 Book 对象 b 的构造函数调用中省略了参数的类型,因为在构造函数定义中已经指明了它们的类型。
8. 在类定义中,修改 char 类型成员变量的 setter 函数,使它们使用 strcpy 函数进行赋值。
阅读全文
相关推荐
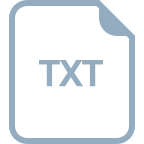
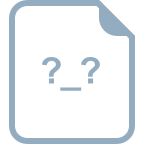
















