int n; printf("请输入节点个数:"); scanf("%d", &n); int* preorder = (int*)malloc(n * sizeof(int)); int* inorder = (int*)malloc(n * sizeof(int)); printf("请按先序遍历序列输入各节点的值:"); for (int i = 0; i < n; i++) { scanf("%d", &preorder[i]); } printf("请按中序遍历序列输入各节点的值:"); for (int i = 0; i < n; i++) { scanf("%d", &inorder[i]); }请改为直接输入节点的值
时间: 2023-07-16 16:11:57 浏览: 66
int n;
printf("请输入节点个数:");
scanf("%d", &n);
int* preorder = (int*)malloc(n * sizeof(int));
int* inorder = (int*)malloc(n * sizeof(int));
printf("请按先序遍历序列输入各节点的值:");
for (int i = 0; i < n; i++) {
scanf("%d", &preorder[i]);
}
printf("请按中序遍历序列输入各节点的值:");
for (int i = 0; i < n; i++) {
scanf("%d", &inorder[i]);
}
// 改为直接输入节点的值
printf("请按顺序输入各节点的值:");
for (int i = 0; i < n; i++) {
scanf("%d", &preorder[i]);
inorder[i] = preorder[i];
}
相关问题
#include <stdio.h> #include <stdlib.h> #define LEN sizeof(tree) typedef struct BiTNode { int data; struct BiTNode* lchild; struct BiTNode* rchild; } tree; void CreatTree(tree** root) { int t; scanf("%d", &t); if (t == 0) { *root = NULL; return; } else { *root = (tree*)malloc(LEN); (*root)->data = t; printf("\n输入%d的左孩子:", (*root)->data); CreatTree(&((*root)->lchild)); printf("输入%d的右孩子:", (*root)->data); CreatTree(&((*root)->rchild)); }}void ClearTree(tree** root) { if (*root == NULL) return; else { ClearTree(&((*root)->lchild)); ClearTree(&((*root)->rchild)); free(*root); *root = NULL; }}// 先序遍历 void preorder(tree* root) { if (root == NULL) return; else { printf("%d ", root->data); preorder(root->lchild); preorder(root->rchild); }}// 中序遍历 void inorder(tree* root) { if (root == NULL) return; else { inorder(root->lchild); printf("%d ", root->data); inorder(root->rchild); }}// 后序遍历 void postorder(tree* root) { if (root == NULL) return; else { postorder(root->lchild); postorder(root->rchild); printf("%d ", root->data); }}int main() { tree* root = NULL; printf("输入根节点:"); CreatTree(&root); printf("%d ", root->data); printf("先序遍历:"); preorder(root); printf("\n中序遍历:"); inorder(root); printf("\n后序遍历:"); postorder(root); ClearTree(&root); return 0;}用c语言编写
这段代码是一个二叉树的创建和遍历程序,使用了C语言的动态内存分配和递归实现遍历功能。
```c
#include <stdio.h>
#include <stdlib.h>
#define LEN sizeof(tree)
typedef struct BiTNode {
int data;
struct BiTNode* lchild;
struct BiTNode* rchild;
} tree;
void CreatTree(tree** root) {
int t;
scanf("%d", &t);
if (t == 0) {
*root = NULL;
return;
}
else {
*root = (tree*)malloc(LEN);
(*root)->data = t;
printf("\n输入%d的左孩子:", (*root)->data);
CreatTree(&((*root)->lchild));
printf("输入%d的右孩子:", (*root)->data);
CreatTree(&((*root)->rchild));
}
}
void ClearTree(tree** root) {
if (*root == NULL)
return;
else {
ClearTree(&((*root)->lchild));
ClearTree(&((*root)->rchild));
free(*root);
*root = NULL;
}
}
// 先序遍历
void preorder(tree* root) {
if (root == NULL)
return;
else {
printf("%d ", root->data);
preorder(root->lchild);
preorder(root->rchild);
}
}
// 中序遍历
void inorder(tree* root) {
if (root == NULL)
return;
else {
inorder(root->lchild);
printf("%d ", root->data);
inorder(root->rchild);
}
}
// 后序遍历
void postorder(tree* root) {
if (root == NULL)
return;
else {
postorder(root->lchild);
postorder(root->rchild);
printf("%d ", root->data);
}
}
int main() {
tree* root = NULL;
printf("输入根节点:");
CreatTree(&root);
printf("%d ", root->data);
printf("先序遍历:");
preorder(root);
printf("\n中序遍历:");
inorder(root);
printf("\n后序遍历:");
postorder(root);
ClearTree(&root);
return 0;
}
```
#include <stdio.h> #include <stdlib.h> #define MAX_N 100 typedef struct TreeNode { char val; struct TreeNode *left; struct TreeNode *right; } TreeNode; int findIdx(char *arr, int start, int end, char val) { for (int i = start; i <= end; i++) { if (arr[i] == val) { return i; } } return -1; } TreeNode *buildTree(char *preorder, char *inorder, int start, int end) { static int preIdx = 0; if (start > end) { return NULL; } TreeNode *node = (TreeNode *)malloc(sizeof(TreeNode)); node->val = preorder[preIdx++]; if (start == end) { node->left = NULL; node->right = NULL; return node; } int inIdx = findIdx(inorder, start, end, node->val); node->left = buildTree(preorder, inorder, start, inIdx - 1); node->right = buildTree(preorder, inorder, inIdx + 1, end); return node; } int getNodeCount(TreeNode *root) { if (root == NULL) { return 0; } return getNodeCount(root->left) + getNodeCount(root->right) + 1; } void printLevelOrder(TreeNode *root) { if (root == NULL) { return; } TreeNode *queue[MAX_N]; int front = 0, rear = 0; queue[rear++] = root; while (front < rear) { int levelSize = rear - front; for (int i = 0; i < levelSize; i++) { TreeNode *node = queue[front++]; printf("%c ", node->val); if (node->left) { queue[rear++] = node->left; } if (node->right) { queue[rear++] = node->right; } } printf("\n"); } } int getChildCount(TreeNode *node) { if (!node || (!node->left && !node->right)) { return 0; } int count = 0; if (node->left) { count++; } if (node->right) { count++; } return count; } int main() { char preorder[MAX_N], inorder[MAX_N], target; int n, len; printf("请输入二叉树长度、先序序列、中序序列:\n"); scanf("%d%s%s", &n, preorder, inorder); len = strlen(preorder); TreeNode *root = buildTree(preorder, inorder, 0, len - 1); printf("层序遍历:\n"); printLevelOrder(root); printf("节点个数为:%d\n", getNodeCount(root)); printf("请输入要查询子节点的节点:\n"); scanf(" %c", &target); TreeNode *node = root; while (node && node->val != target) { if (node->val > target) { node = node->left; } else { node = node->right; } } if (!node) { printf("未找到该节点!\n"); } else { printf("子节点个数为:%d\n", getChildCount(node)); } return 0; }
这段代码是一段用 C 语言写的二叉树的操作程序。它实现了二叉树的构建、节点数量的统计、层序遍历、以及查询某个节点的子节点数量。其中,buildTree 函数用先序遍历和中序遍历序列构建二叉树,getNodeCount 函数用递归的方式统计二叉树的节点数量,printLevelOrder 函数用队列实现二叉树的层序遍历,getChildCount 函数用来查询某个节点的子节点数量。这段程序还包含了一些输入输出的操作,例如读入二叉树的长度和先序、中序序列,以及输出层序遍历结果和节点数量。
阅读全文
相关推荐
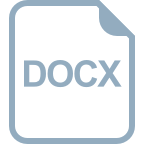
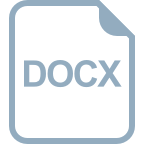
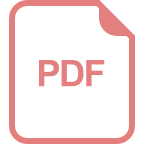













