c语言代码 对于二叉树的输入,空用“^”表示。 首先输入一个二叉树。然后输出: 先序遍历结果“=:先序遍历结果回车”; 中序遍历结果“=:中序遍历结果回车”; 后序遍历结果“=:后序遍历结果回车”; 层序遍历结果“=:层序遍历结果回车”; 叶子数“=:叶子数” 树的深度“=:树的深度回车”。
时间: 2023-08-11 18:05:46 浏览: 85
以下是基于 C 语言的二叉树遍历和统计叶子节点数和深度的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点结构体
typedef struct TreeNode {
char data;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 创建二叉树
TreeNode* createTree() {
char c;
scanf("%c", &c);
if (c == '^') {
return NULL;
}
TreeNode *root = (TreeNode*)malloc(sizeof(TreeNode));
root->data = c;
root->left = createTree();
root->right = createTree();
return root;
}
// 先序遍历
void preOrder(TreeNode *root) {
if (root == NULL) {
return;
}
printf("%c ", root->data);
preOrder(root->left);
preOrder(root->right);
}
// 中序遍历
void inOrder(TreeNode *root) {
if (root == NULL) {
return;
}
inOrder(root->left);
printf("%c ", root->data);
inOrder(root->right);
}
// 后序遍历
void postOrder(TreeNode *root) {
if (root == NULL) {
return;
}
postOrder(root->left);
postOrder(root->right);
printf("%c ", root->data);
}
// 层序遍历
void levelOrder(TreeNode *root) {
if (root == NULL) {
return;
}
TreeNode *queue[1000];
int front = 0, rear = 0;
queue[rear++] = root;
while (front != rear) {
TreeNode *temp = queue[front++];
printf("%c ", temp->data);
if (temp->left != NULL) {
queue[rear++] = temp->left;
}
if (temp->right != NULL) {
queue[rear++] = temp->right;
}
}
}
// 统计叶子节点数
int countLeaf(TreeNode *root) {
if (root == NULL) {
return 0;
}
if (root->left == NULL && root->right == NULL) {
return 1;
}
return countLeaf(root->left) + countLeaf(root->right);
}
// 计算树的深度
int depth(TreeNode *root) {
if (root == NULL) {
return 0;
}
int leftDepth = depth(root->left);
int rightDepth = depth(root->right);
return leftDepth > rightDepth ? leftDepth + 1 : rightDepth + 1;
}
int main() {
printf("请输入二叉树,用^表示空:\n");
TreeNode *root = createTree();
printf("\n先序遍历结果=");
preOrder(root);
printf("\n中序遍历结果=");
inOrder(root);
printf("\n后序遍历结果=");
postOrder(root);
printf("\n层序遍历结果=");
levelOrder(root);
printf("\n叶子数=%d\n", countLeaf(root));
printf("树的深度=%d\n", depth(root));
return 0;
}
```
注意事项:
1. 输入时请保证二叉树的形式正确,即左右子树都用 '^' 表示为空。
2. 叶子节点数的计算方法为:左右子树都为空时为 1,否则为左子树叶子节点数加上右子树叶子节点数之和。
3. 深度的计算方法为:左右子树深度较大的值加上 1。
阅读全文
相关推荐
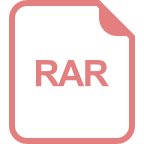
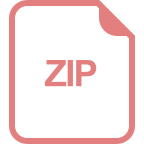















