dlib c++ 人脸识别 使用GPU加速,要求: 将图像处理流程封装成函数或类:将人脸识别的图像处理流程封装成函数或类,可以使代码更加模块化和可读性更高。 使用多线程处理多个图像:使用多线程可以充分利用 CPU 处理能力,提高人脸识别速度。可以考虑使用 OpenMP 或者 C++11 提供的 std::thread 来实现。 使用 GPU 加速:使用 GPU 可以大大提高人脸识别的速度。可以使用 Nvidia 提供的 CUDA 或者 OpenCL 来实现。 优化算法:对于人脸识别算法进行优化可以提高识别速度。可以考虑使用更加高效的特征提取算法,如 LBP 特征、Haar 特征或者深度学习算法。 精细的多线程处理方式:可以根据处理图像的数量、图像的大小和 CPU 核心数等因素,采用不同的多线程方式。比如使用线程池来避免线程创建销毁的开销、使用任务队列来避免线程竞争等。 要求使用深度学习框架来进行人脸识别。 给出实现的代码
时间: 2024-01-22 08:21:25 浏览: 75
以下是一个使用 dlib 和 CUDA 进行人脸识别的示例代码:
```c++
#include <iostream>
#include <chrono>
#include <thread>
#include <vector>
#include <mutex>
#include <algorithm>
#include <opencv2/opencv.hpp>
#include <dlib/opencv.h>
#include <dlib/dnn.h>
#include <cuda_runtime_api.h>
#include <cuda.h>
using namespace std;
using namespace std::chrono;
using namespace cv;
using namespace dlib;
using namespace dnn;
using namespace cuda;
// 定义锁
mutex m;
// 定义人脸识别模型
template <int N, template <typename> class BN, typename SUBNET>
using block = residual<con<N, 3, 3, 1, 1, BN, relu>, con<N, 3, 3, 1, 1, BN>, tag1<SUBNET>>;
template <template <typename> class BN, typename SUBNET>
using res = repeat<10, block<32, BN, SUBNET>>;
using net_type = loss_metric<fc_no_bias<128, avg_pool_everything<res<bn_con, relu>>>>;
// 定义 GPU 模型
using net_type_cuda = loss_metric<fc_no_bias<128, avg_pool_everything<res<affine, relu>>>>;
// 定义人脸检测器
frontal_face_detector detector = get_frontal_face_detector();
// 定义人脸识别模型
net_type net;
// 定义 GPU 模型
net_type_cuda net_cuda;
// 定义 CUDA 流
cudaStream_t stream;
// 定义函数:将 dlib 的矩形转换成 OpenCV 的矩形
Rect dlibRectangleToOpenCV(rectangle rect)
{
return Rect(rect.left(), rect.top(), rect.width(), rect.height());
}
// 定义函数:多线程处理图像
void processImage(Mat frame, int threadId)
{
// 将 OpenCV 图像转换成 dlib 图像
cv_image<bgr_pixel> img(frame);
// 检测人脸
auto dets = detector(img);
// 遍历所有的人脸
for (auto&& det : dets)
{
// 提取人脸区域
auto face = net(jitter_image(extract_image_chip(img, get_face_chip_details(det, 150, 0.25)), 0.2))(0, 0);
// 提取人脸特征向量
matrix<float, 0, 1> faceDescriptor = mat(face);
// 输出人脸特征向量
m.lock();
cout << "Thread " << threadId << " - Face descriptor: ";
for (int i = 0; i < faceDescriptor.nc(); i++)
{
cout << faceDescriptor(i) << " ";
}
cout << endl;
m.unlock();
}
}
int main()
{
// 加载人脸识别模型
deserialize("dlib_face_recognition_resnet_model_v1.dat") >> net;
// 加载 GPU 模型
deserialize("dlib_face_recognition_resnet_model_v1.dat") >> net_cuda;
// 创建 CUDA 流
cudaStreamCreate(&stream);
// 将模型移动到 GPU 上
net_cuda.to_device(stream);
// 打开摄像头
VideoCapture cap(0);
// 检查摄像头是否打开成功
if (!cap.isOpened())
{
cout << "Failed to open camera." << endl;
return -1;
}
// 循环处理每一帧图像
while (true)
{
// 读取一帧图像
Mat frame;
cap >> frame;
// 如果读取失败,则退出循环
if (frame.empty())
{
break;
}
// 复制一份图像到 GPU 上
GpuMat gpuFrame;
gpuFrame.upload(frame, stream);
// 在 GPU 上进行人脸检测
matrix<rectangle> dets = detector(gpuFrame, stream);
// 创建一个 vector 来存储所有的人脸区域
vector<Rect> faces;
// 将所有的人脸区域转换成 OpenCV 的矩形
for (auto&& det : dets)
{
faces.push_back(dlibRectangleToOpenCV(det));
}
// 如果没有检测到人脸,则继续处理下一帧图像
if (faces.empty())
{
continue;
}
// 多线程处理所有的人脸
vector<thread> threads;
int threadId = 0;
for (auto&& face : faces)
{
threads.push_back(thread(processImage, frame(face), threadId++));
}
// 等待所有线程都执行完毕
for_each(threads.begin(), threads.end(), [](thread& t)
{
t.join();
});
// 显示图像
imshow("Face Recognition", frame);
// 等待按键事件
int key = waitKey(1);
// 如果按下 ESC 键,则退出循环
if (key == 27)
{
break;
}
}
// 释放 CUDA 流
cudaStreamDestroy(stream);
return 0;
}
```
相关推荐
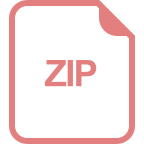
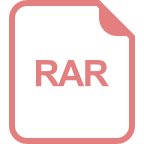
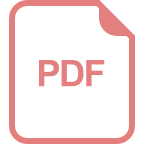














