python打地鼠小游戏的详细设计
时间: 2023-07-27 11:18:38 浏览: 126
好的,下面是Python打地鼠小游戏的详细设计说明:
1. 导入必要的库和初始化游戏
在程序的开头,我们需要导入pygame和其他必要的库,并初始化pygame和游戏屏幕:
```python
import pygame
import random
pygame.init()
# 设置屏幕大小
screen_width = 640
screen_height = 480
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("打地鼠小游戏")
```
2. 加载游戏所需的图像
我们需要加载游戏所需的图像,如背景图,地鼠图等:
```python
# 加载背景图
background_img = pygame.image.load("background.png").convert()
# 加载地鼠图和地洞图
hole_img = pygame.image.load("hole.png").convert_alpha()
mole_imgs = []
for i in range(1, 4):
mole_img = pygame.image.load(f"mole{i}.png").convert_alpha()
mole_imgs.append(mole_img)
```
3. 创建地鼠类和洞类
我们需要创建地鼠类和洞类,并定义它们的行为:
```python
class Hole(pygame.sprite.Sprite):
def __init__(self, position):
super().__init__()
self.image = hole_img
self.rect = self.image.get_rect()
self.rect.topleft = position
def get_random_pos(self):
# 获取地洞的随机位置
x = self.rect.left + 30
y = self.rect.top + 30
return random.randint(x, x + 100), random.randint(y, y + 50)
class Mole(pygame.sprite.Sprite):
def __init__(self, position):
super().__init__()
self.image = mole_imgs[0]
self.rect = self.image.get_rect()
self.rect.topleft = position
self.animation_frames = mole_imgs
self.current_frame = 0
self.show_time = 20
self.hide_time = 40
self.current_time = 0
self.is_showing = False
def update(self):
# 更新地鼠的状态
self.current_time += 1
if self.is_showing:
if self.current_time >= self.show_time:
self.current_time = 0
self.is_showing = False
self.image = self.animation_frames[0]
else:
if self.current_time >= self.hide_time:
self.current_time = 0
self.is_showing = True
self.image = random.choice(self.animation_frames)
```
4. 定义游戏的主循环,并在其中实现游戏的逻辑
我们需要定义游戏的主循环,并在其中实现游戏的逻辑:
```python
def main():
# 创建地洞和地鼠组
hole_group = pygame.sprite.Group()
mole_group = pygame.sprite.Group()
for i in range(3):
hole = Hole((i * 200 + 50, 200))
hole_group.add(hole)
mole = Mole(hole.get_random_pos())
mole_group.add(mole)
# 设置游戏时钟和字体
clock = pygame.time.Clock()
font = pygame.font.Font(None, 36)
# 初始化游戏状态
score = 0
time_left = 30 * 1000
game_over = False
# 游戏循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
# 处理玩家点击事件
pos = pygame.mouse.get_pos()
for mole in mole_group:
if mole.rect.collidepoint(pos) and mole.is_showing:
score += 10
mole.is_showing = False
mole.current_time = 0
mole.image = mole.animation_frames[0]
# 更新游戏状态
time_left -= clock.tick(30)
if time_left <= 0:
game_over = True
else:
mole_group.update()
# 绘制游戏界面
screen.blit(background_img, (0, 0))
hole_group.draw(screen)
mole_group.draw(screen)
score_text = font.render(f"得分:{score}", True, (255, 255, 255))
screen.blit(score_text, (10, 10))
time_text = font.render(f"时间:{int(time_left / 1000)}", True, (255, 255, 255))
screen.blit(time_text, (screen_width - time_text.get_width() - 10, 10))
if game_over:
game_over_text = font.render("游戏结束!", True, (255, 0, 0))
screen.blit(game_over_text, (screen_width // 2 - game_over_text.get_width() // 2, screen_height // 2 - game_over_text.get_height() // 2))
pygame.display.update()
```
5. 运行游戏
最后,我们需要在程序的末尾调用main()函数来运行游戏:
```python
if __name__ == "__main__":
main()
```
以上就是Python打地鼠小游戏的详细设计说明。您可以根据这些说明来编写自己的打地鼠小游戏。
阅读全文
相关推荐















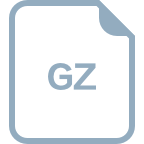