python json dump后格式
时间: 2023-10-21 18:08:59 浏览: 154
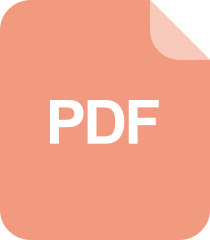
python数据封装json格式数据
在 Python 中,使用 `json.dump()` 方法将数据转换为 JSON 格式后,其格式默认为紧凑格式,即没有空格或换行符。如果要使输出的 JSON 字符串更易读,可以添加参数 `indent` 来指定缩进的空格数量。例如:
```python
import json
data = {"name": "Alice", "age": 25, "city": "New York"}
# 使用默认格式输出
json_str = json.dumps(data)
print(json_str)
# 输出:{"name": "Alice", "age": 25, "city": "New York"}
# 使用缩进格式输出
json_str = json.dumps(data, indent=4)
print(json_str)
# 输出:
# {
# "name": "Alice",
# "age": 25,
# "city": "New York"
# }
```
在上面的例子中,`json.dumps()` 方法的第二个参数 `indent` 被设置为 4,指定了输出的 JSON 字符串使用 4 个空格作为缩进。
阅读全文