python两个一维数组先叉乘再点乘
时间: 2023-07-26 14:44:29 浏览: 65
可以使用numpy库实现两个一维数组的叉乘和点乘。
叉乘可以使用numpy的cross函数实现:
```python
import numpy as np
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
c = np.cross(a, b)
print(c)
```
输出结果为:
```
[-3 6 -3]
```
点乘可以使用numpy的dot函数实现:
```python
import numpy as np
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
c = np.dot(a, b)
print(c)
```
输出结果为:
```
32
```
需要注意的是,叉乘的结果是一个向量,而点乘的结果是一个标量。
相关问题
python数组的乘法
回答: 在Python中,数组的乘法有两种不同的含义。一种是点乘,也称为元素级乘法,使用符号*表示。这种乘法是对应元素相乘,即数组中的每个元素与另一个数组中相同位置的元素相乘,得到一个新的数组。例如,如果有两个数组A和B,A * B将返回一个新的数组,其中每个元素是A和B对应位置元素的乘积。\[1\]
另一种是矩阵乘法,使用函数dot()或@符号表示。这种乘法是对两个数组进行矩阵乘法运算,得到一个新的数组。矩阵乘法要求两个数组的维度满足矩阵乘法的规则,即第一个数组的列数等于第二个数组的行数。例如,如果有两个二维数组A和B,dot(A, B)或A @ B将返回一个新的数组,其中每个元素是A和B进行矩阵乘法运算后的结果。\[2\]
需要注意的是,使用*进行的点乘操作是对应元素相乘,而不是矩阵乘法。如果想要进行矩阵乘法运算,应该使用dot()函数或@符号。\[1\]\[2\]
#### 引用[.reference_title]
- *1* [python数组(矩阵)乘法(点乘、叉乘)](https://blog.csdn.net/qq_32649321/article/details/123012420)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [Python+Numpy------数组运算](https://blog.csdn.net/liyuqian199695/article/details/53863173)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
设计一个三维向量类Vector3D,包含三维向量的一些基本运算,如加法、减法以及点乘、叉乘。
好的,下面是一个简单的 Vector3D 类的示例代码:
```python
class Vector3D:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
def __add__(self, other):
return Vector3D(self.x + other.x, self.y + other.y, self.z + other.z)
def __sub__(self, other):
return Vector3D(self.x - other.x, self.y - other.y, self.z - other.z)
def dot_product(self, other):
return self.x * other.x + self.y * other.y + self.z * other.z
def cross_product(self, other):
return Vector3D(self.y * other.z - self.z * other.y,
self.z * other.x - self.x * other.z,
self.x * other.y - self.y * other.x)
```
这个类包含了一个构造函数和四个方法:
- `__init__(self, x, y, z)`:构造函数,用于初始化向量的三个分量。
- `__add__(self, other)`:重载加法运算符,用于向量的加法。
- `__sub__(self, other)`:重载减法运算符,用于向量的减法。
- `dot_product(self, other)`:点乘方法,用于计算两个向量的点乘积。
- `cross_product(self, other)`:叉乘方法,用于计算两个向量的叉乘积。
使用示例:
```python
# 创建两个向量
v1 = Vector3D(1, 2, 3)
v2 = Vector3D(4, 5, 6)
# 向量加法
v3 = v1 + v2
print(v3.x, v3.y, v3.z) # 输出:5 7 9
# 向量减法
v4 = v2 - v1
print(v4.x, v4.y, v4.z) # 输出:3 3 3
# 向量点乘
dot_product = v1.dot_product(v2)
print(dot_product) # 输出:32
# 向量叉乘
v5 = v1.cross_product(v2)
print(v5.x, v5.y, v5.z) # 输出:(-3, 6, -3)
```
相关推荐











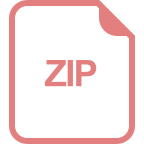
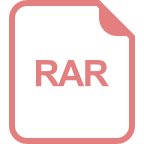