springboot对新闻控制层的单元测试代码实现
时间: 2023-07-26 22:51:49 浏览: 39
好的,以下是一个简单的示例代码,用于展示如何使用Spring Boot Test和MockMvc来编写新闻控制层的单元测试:
```java
@RunWith(SpringRunner.class)
@SpringBootTest
@AutoConfigureMockMvc
public class NewsControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testGetNewsList() throws Exception {
mockMvc.perform(get("/news"))
.andExpect(status().isOk())
.andExpect(jsonPath("$", hasSize(2)))
.andExpect(jsonPath("$[0].title", is("News 1")))
.andExpect(jsonPath("$[1].title", is("News 2")));
}
@Test
public void testCreateNews() throws Exception {
News news = new News();
news.setTitle("News 3");
news.setContent("This is news 3.");
mockMvc.perform(post("/news")
.contentType(MediaType.APPLICATION_JSON)
.content(new ObjectMapper().writeValueAsString(news)))
.andExpect(status().isOk())
.andExpect(jsonPath("$.title", is("News 3")))
.andExpect(jsonPath("$.content", is("This is news 3.")));
}
@Test
public void testUpdateNews() throws Exception {
News news = new News();
news.setTitle("News 2 Updated");
news.setContent("This is news 2 updated.");
mockMvc.perform(put("/news/{id}", 2)
.contentType(MediaType.APPLICATION_JSON)
.content(new ObjectMapper().writeValueAsString(news)))
.andExpect(status().isOk())
.andExpect(jsonPath("$.title", is("News 2 Updated")))
.andExpect(jsonPath("$.content", is("This is news 2 updated.")));
}
@Test
public void testDeleteNews() throws Exception {
mockMvc.perform(delete("/news/{id}", 1))
.andExpect(status().isOk());
}
}
```
在这个示例中,我们使用了Spring Boot Test和MockMvc来模拟HTTP请求,然后对新闻控制层的四个接口进行了测试。具体来说:
- testGetNewsList()方法测试了获取新闻列表的接口,验证了响应的状态码、响应的JSON数组长度以及每个新闻的标题是否正确。
- testCreateNews()方法测试了创建新闻的接口,使用了一个News对象作为请求体,验证了响应的状态码以及响应的JSON对象中的标题和内容是否正确。
- testUpdateNews()方法测试了更新新闻的接口,同样使用了一个News对象作为请求体,验证了响应的状态码以及响应的JSON对象中的标题和内容是否正确。
- testDeleteNews()方法测试了删除新闻的接口,验证了响应的状态码是否正确。
总之,这个示例代码演示了如何使用Spring Boot Test和MockMvc来编写新闻控制层的单元测试,并对每个接口进行了简单的测试验证。
相关推荐
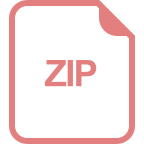
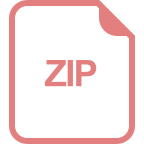
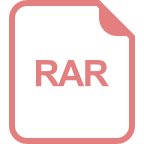














