使用c写一个excel转json代码
时间: 2024-02-03 08:11:18 浏览: 18
以下是一个简单的Excel转换为JSON格式的示例代码。注意,此代码假定Excel文件的第一行是列标题,第二行及以下是数据行。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_ROWS 1000
#define MAX_COLS 100
char buffer[1024*1024];
int main(int argc, char **argv) {
if (argc != 3) {
printf("Usage: excel2json inputfile outputfile\n");
return 1;
}
char *infile = argv[1];
char *outfile = argv[2];
FILE *fp = fopen(infile, "r");
if (!fp) {
printf("Error: cannot open file %s\n", infile);
return 1;
}
char line[1024];
int row_count = 0;
int col_count = 0;
char *cols[MAX_COLS];
// Read column headers
if (fgets(line, sizeof(line), fp)) {
char *p = strtok(line, "\t\r\n");
while (p) {
cols[col_count++] = strdup(p);
p = strtok(NULL, "\t\r\n");
}
}
// Read data rows
while (fgets(line, sizeof(line), fp)) {
if (row_count >= MAX_ROWS) {
printf("Error: too many rows\n");
return 1;
}
int col_index = 0;
char *p = strtok(line, "\t\r\n");
while (p && col_index < col_count) {
sprintf(buffer, "\"%s\":\"%s\",", cols[col_index++], p);
strcat(buffer, "\n");
fwrite(buffer, strlen(buffer), 1, stdout);
p = strtok(NULL, "\t\r\n");
}
row_count++;
}
// Write JSON output to file
FILE *out = fopen(outfile, "w");
if (!out) {
printf("Error: cannot create file %s\n", outfile);
return 1;
}
fprintf(out, "{\n");
for (int i = 0; i < col_count; i++) {
sprintf(buffer, "\"%s\": [", cols[i]);
strcat(buffer, "\n");
fwrite(buffer, strlen(buffer), 1, out);
fseek(fp, 0, SEEK_SET);
// Skip header row
fgets(line, sizeof(line), fp);
int row_index = 0;
while (fgets(line, sizeof(line), fp)) {
char *p = strtok(line, "\t\r\n");
for (int j = 0; j < i; j++) {
p = strtok(NULL, "\t\r\n");
}
if (row_index > 0) {
fwrite(",", 1, 1, out);
fwrite("\n", 1, 1, out);
}
if (p && *p) {
sprintf(buffer, "\"%s\"", p);
fwrite(buffer, strlen(buffer), 1, out);
}
row_index++;
}
fwrite("\n", 1, 1, out);
fwrite("],\n", 2, 2, out);
}
fprintf(out, "}\n");
fclose(fp);
fclose(out);
return 0;
}
```
这个程序将Excel文件转换为JSON格式,并将结果写入输出文件中。要使用该程序,请键入以下命令:
```
excel2json inputfile.xlsx outputfile.json
```
其中 `inputfile.xlsx` 是要转换的Excel文件,`outputfile.json` 是输出的JSON文件。
相关推荐
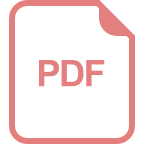
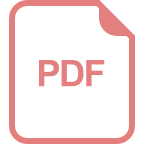
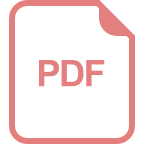














