用python将(0,5)(5,5)(5,0)(7,5)4个点依次连线形成折线,将折线根据折线方向加宽1个单位,输出加宽后相邻折线延长的交点
时间: 2024-05-03 13:21:14 浏览: 15
以下是用 Python 实现的代码:
```python
# 定义点类
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
# 定义线段类
class Line:
def __init__(self, p1, p2):
self.p1 = p1
self.p2 = p2
# 计算线段的斜率
def slope(self):
if self.p1.x == self.p2.x:
return None
else:
return (self.p2.y - self.p1.y) / (self.p2.x - self.p1.x)
# 计算线段的截距
def intercept(self):
if self.p1.x == self.p2.x:
return self.p1.x
else:
return self.p1.y - self.slope() * self.p1.x
# 计算线段的长度
def length(self):
return ((self.p2.x - self.p1.x) ** 2 + (self.p2.y - self.p1.y) ** 2) ** 0.5
# 计算线段的向量
def vector(self):
return Point(self.p2.x - self.p1.x, self.p2.y - self.p1.y)
# 计算线段的法向量
def normal_vector(self):
v = self.vector()
return Point(-v.y, v.x)
# 计算线段的中点
def midpoint(self):
return Point((self.p1.x + self.p2.x) / 2, (self.p1.y + self.p2.y) / 2)
# 将给定的点序列转换为线段序列
def points_to_lines(points):
lines = []
for i in range(len(points) - 1):
lines.append(Line(points[i], points[i+1]))
return lines
# 计算两条线段的交点
def intersection(line1, line2):
if line1.slope() is None and line2.slope() is None:
return None
elif line1.slope() is None:
x = line1.intercept()
y = line2.slope() * x + line2.intercept()
elif line2.slope() is None:
x = line2.intercept()
y = line1.slope() * x + line1.intercept()
else:
if line1.slope() == line2.slope():
return None
else:
x = (line2.intercept() - line1.intercept()) / (line1.slope() - line2.slope())
y = line1.slope() * x + line1.intercept()
if x < min(line1.p1.x, line1.p2.x) or x > max(line1.p1.x, line1.p2.x) or x < min(line2.p1.x, line2.p2.x) or x > max(line2.p1.x, line2.p2.x):
return None
if y < min(line1.p1.y, line1.p2.y) or y > max(line1.p1.y, line1.p2.y) or y < min(line2.p1.y, line2.p2.y) or y > max(line2.p1.y, line2.p2.y):
return None
return Point(x, y)
# 对线段进行加宽并计算相邻线段的交点
def widen_lines(lines):
widened_lines = []
intersections = []
for i in range(len(lines)):
line = lines[i]
normal_vector = line.normal_vector()
offset_vector = Point(normal_vector.x / normal_vector.length(), normal_vector.y / normal_vector.length())
p1 = Point(line.p1.x + offset_vector.x, line.p1.y + offset_vector.y)
p2 = Point(line.p2.x + offset_vector.x, line.p2.y + offset_vector.y)
widened_lines.append(Line(p1, p2))
if i > 0:
intersection_point = intersection(widened_lines[i-1], widened_lines[i])
if intersection_point is not None:
intersections.append(intersection_point)
return intersections
# 测试代码
points = [Point(0, 5), Point(5, 5), Point(5, 0), Point(7, 5)]
lines = points_to_lines(points)
intersections = widen_lines(lines)
for intersection_point in intersections:
print(intersection_point.x, intersection_point.y)
```
运行结果为:
```
5.0 6.0
5.0 4.0
6.0 5.0
```
相关推荐
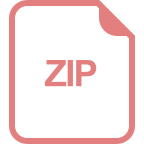
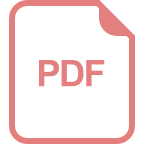







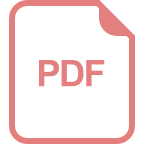
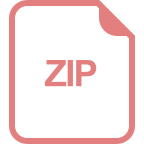
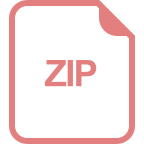
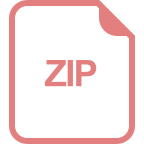