import numpy as np # 定义4x2矩阵A A = np.array([[1, 2], [3, 4], [5, 6], [7, 8]]) # 对A进行奇异值分解 U, S, V_T = np.linalg.svd(A) # 构造正交矩阵B B = U.dot(V_T) # 构造矩阵C C = np.array([[np.sqrt(1 - S[1]**2), S[1]], [S[1], np.sqrt(1 - S[1]**2)]]) print("矩阵A:") print(A) print("正交矩阵B:") print(B) print("矩阵C:") print(C)如何将A,B,C都变成复数矩阵
时间: 2023-10-31 09:05:17 浏览: 208
可以使用numpy的conjugate函数将实数矩阵转换为复数矩阵。具体实现如下:
```
import numpy as np
# 定义4x2矩阵A
A = np.array([[1, 2], [3, 4], [5, 6], [7, 8]])
# 对A进行奇异值分解
U, S, V_T = np.linalg.svd(A)
# 构造正交矩阵B
B = U.dot(V_T)
# 构造矩阵C
C = np.array([[np.sqrt(1 - S[1]**2), S[1]], [S[1], np.sqrt(1 - S[1]**2)]])
# 将A,B,C都变成复数矩阵
A_complex = A.astype(np.complex128)
B_complex = B.astype(np.complex128)
C_complex = np.array([[np.sqrt(1 - S[1]**2), S[1]], [S[1], np.sqrt(1 - S[1]**2)]]).astype(np.complex128)
print("复数矩阵A:")
print(A_complex)
print("复数正交矩阵B:")
print(B_complex)
print("复数矩阵C:")
print(C_complex)
```
注意,需要将矩阵元素的数据类型转换为np.complex128。
相关问题
import numpy as np array = np.arange(4) Z = np.diag(1+array, k=-1) print(Z)解释一下
这段代码是用NumPy库创建一个4x4的矩阵Z,其中对角线上的元素为1,其余元素均为0,且在对角线下方一格的位置也填充了1。具体实现是通过np.diag()函数创建一个以1为对角线元素、在对角线下方一格填充1的二维数组,然后将其赋值给Z并打印输出。
import numpy as np class BPNeuralNetwork: def __init__(self, input_size, hidden_size, output_size): self.input_size = input_size self.hidden_size = hidden_size self.output_size = output_size self.weights1 = np.random.randn(self.input_size, self.hidden_size) self.bias1 = np.zeros((1, self.hidden_size)) self.weights2 = np.random.randn(self.hidden_size, self.output_size) self.bias2 = np.zeros((1, self.output_size)) def forward(self, X): self.hidden_layer = np.dot(X, self.weights1) + self.bias1 self.activated_hidden_layer = self.sigmoid(self.hidden_layer) self.output_layer = np.dot(self.activated_hidden_layer, self.weights2) + self.bias2 self.activated_output_layer = self.sigmoid(self.output_layer) return self.activated_output_layer def sigmoid(self, s): return 1 / (1 + np.exp(-s)) def sigmoid_derivative(self, s): return s * (1 - s) def backward(self, X, y, o, learning_rate): self.error = y - o self.delta_output = self.error * self.sigmoid_derivative(o) self.error_hidden = self.delta_output.dot(self.weights2.T) self.delta_hidden = self.error_hidden * self.sigmoid_derivative(self.activated_hidden_layer) self.weights1 += X.T.dot(self.delta_hidden) * learning_rate self.bias1 += np.sum(self.delta_hidden, axis=0, keepdims=True) * learning_rate self.weights2 += self.activated_hidden_layer.T.dot(self.delta_output) * learning_rate self.bias2 += np.sum(self.delta_output, axis=0, keepdims=True) * learning_rate def train(self, X, y, learning_rate, epochs): for epoch in range(epochs): output = self.forward(X) self.backward(X, y, output, learning_rate) def predict(self, X): return self.forward(X) X = np.array([[0, 0, 1], [0, 1, 1], [1, 0, 1], [1, 1, 1]]) y = np.array([[0], [1], [1], [0]]) nn = BPNeuralNetwork(3, 4, 1) nn.train(X, y, 0.1, 10000) new_data = np.array([[0, 0, 0], [0, 1, 0], [1, 0, 0], [1, 1, 0]]) print(nn.predict(new_data))
这是一个使用反向传播算法实现的简单的三层神经网络,输入层有3个节点,隐藏层有4个节点,输出层有1个节点。它的训练数据X是一个4x3的矩阵,y是一个4x1的矩阵。训练过程中,使用随机初始化的权重和偏置,对训练数据进行前向传播,计算输出值,在计算误差,并使用反向传播算法更新权重和偏置,最终得到一个可以进行二分类的神经网络模型。
在代码的最后,我们使用训练好的模型对新数据进行了预测,输出预测的结果。
阅读全文
相关推荐
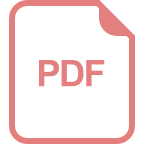
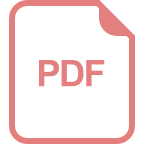
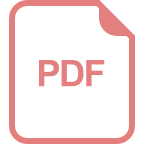














