使用c++编写qt时,如何将vector<string>强转为void*传递地址,再强转为vector<string>使用
时间: 2024-03-20 08:43:39 浏览: 163
将 `vector<string>` 强制转换为 `void*` 是不安全的,因为 `vector<string>` 是一个类,它有很多成员变量和成员函数,强制转换后会丢失这些信息,导致程序出错。正确的做法是使用 `reinterpret_cast` 将 `vector<string>` 的地址转换为 `void*`,再在需要使用的地方将 `void*` 转换回 `vector<string>`。
示例代码如下:
```
// 将 vector<string> 转换为 void*
vector<string> strVec;
void* pData = reinterpret_cast<void*>(&strVec);
// 在需要使用的地方将 void* 转换回 vector<string>
void* pTemp = getData(); // 假设 getData() 返回 void*
vector<string> tempVec = *reinterpret_cast<vector<string>*>(pTemp);
```
需要注意的是,这种类型的转换是危险的,如果你不确定自己在做什么,就应该避免这种转换。
相关问题
qt父窗口mainwindow有两个容器vector<string> delete_pathlists; vector<unsigned char> crosstypelists; 在子窗口deletewindow上显示出来,给出C++完整示例
好的,以下是一个简单的示例:
MainWindow.h:
```cpp
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <vector>
#include <string>
namespace Ui {
class MainWindow;
}
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void on_deleteButton_clicked();
private:
Ui::MainWindow *ui;
std::vector<std::string> delete_pathlists;
std::vector<unsigned char> crosstypelists;
};
#endif // MAINWINDOW_H
```
MainWindow.cpp:
```cpp
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include "deletewindow.h"
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
// 添加一些示例数据
delete_pathlists.push_back("path1");
delete_pathlists.push_back("path2");
delete_pathlists.push_back("path3");
crosstypelists.push_back(1);
crosstypelists.push_back(2);
crosstypelists.push_back(3);
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::on_deleteButton_clicked()
{
// 创建删除窗口并传递数据
DeleteWindow *deleteWindow = new DeleteWindow(this, delete_pathlists, crosstypelists);
deleteWindow->show();
}
```
DeleteWindow.h:
```cpp
#ifndef DELETEWINDOW_H
#define DELETEWINDOW_H
#include <QDialog>
#include <vector>
#include <string>
namespace Ui {
class DeleteWindow;
}
class DeleteWindow : public QDialog
{
Q_OBJECT
public:
explicit DeleteWindow(QWidget *parent = nullptr,
const std::vector<std::string>& delete_pathlists = std::vector<std::string>(),
const std::vector<unsigned char>& crosstypelists = std::vector<unsigned char>());
~DeleteWindow();
private:
Ui::DeleteWindow *ui;
};
#endif // DELETEWINDOW_H
```
DeleteWindow.cpp:
```cpp
#include "deletewindow.h"
#include "ui_deletewindow.h"
DeleteWindow::DeleteWindow(QWidget *parent,
const std::vector<std::string>& delete_pathlists,
const std::vector<unsigned char>& crosstypelists) :
QDialog(parent),
ui(new Ui::DeleteWindow)
{
ui->setupUi(this);
// 在窗口中显示数据
for (const auto& path : delete_pathlists) {
ui->pathList->addItem(QString::fromStdString(path));
}
for (const auto& type : crosstypelists) {
ui->typeList->addItem(QString::number(type));
}
}
DeleteWindow::~DeleteWindow()
{
delete ui;
}
```
在这个示例中,我们在 `MainWindow` 中创建了一个 `vector<string>` 和一个 `vector<unsigned char>`,然后在点击删除按钮时打开了一个 `DeleteWindow` 窗口并将这些数据传递给它,在 `DeleteWindow` 中我们可以将这些数据显示在两个列表中。
#include<iostream> #include<stdio.h> #include<string.h> #include<math.h> #include<algorithm> #include<vector> #include<set> #include<map> #include<string> #include<queue> #include<stack> #include <iomanip> using namespace std; #define LL long long const int INF=0x3f3f3f3f; const int N=106; int head[N],I; struct node { double r,c; int j,next; }side[N*2]; double dist[N]; void add(int i,int j,double r,double c) { side[I].j=j; side[I].c=c; side[I].r=r; side[I].next=head[i]; head[i]=I++; } bool spfa(int s,double k,int n) { int num[N]; bool in[N]; memset(num,0,sizeof(num)); memset(in,false,sizeof(in)); queue<int>qt; for(int i=1;i<=n;++i) dist[i]=0.0; dist[s]=k; qt.push(s); num[s]=1; while(!qt.empty()) { int x=qt.front();qt.pop(); in[x]=false; if(x==s&&dist[x]>k) return true; for(int t=head[x];t!=-1;t=side[t].next) { int j=side[t].j; if(dist[j]<(dist[x]-side[t].c)*side[t].r) { dist[j]=(dist[x]-side[t].c)*side[t].r; while(!in[j]) { ++num[j]; if(num[j]>=n) return true; in[j]=true; qt.push(j); } } } } return false; } int main() { //freopen("data.in","r",stdin); int n,m,s; double k; while(cin>>n>>m>>s>>k) { memset(head,-1,sizeof(head)); I=0; while(m--) { int a,b; double rab,cab,rba,cba; cin>>a>>b>>rab>>cab>>rba>>cba; add(a,b,rab,cab); add(b,a,rba,cba); } if(spfa(s,k,n)) cout<<"YES"<<endl; else cout<<"NO"<<endl; } return 0; }把这段c++改为Java
将这段 C++ 代码翻译为 Java 代码如下:
```
import java.io.*;
import java.util.*;
public class Main {
static class Node {
double r, c;
int j, next;
Node(int j, double r, double c, int next) {
this.j = j;
this.r = r;
this.c = c;
this.next = next;
}
}
static final int INF = 0x3f3f3f3f;
static final int N = 106;
static int[] head = new int[N];
static int I;
static Node[] side = new Node[N * 2];
static double[] dist = new double[N];
static void add(int i, int j, double r, double c) {
side[I] = new Node(j, r, c, head[i]);
head[i] = I++;
}
static boolean spfa(int s, double k, int n) {
int[] num = new int[N];
boolean[] in = new boolean[N];
Arrays.fill(num, 0);
Arrays.fill(in, false);
Queue<Integer> qt = new LinkedList<>();
for (int i = 1; i <= n; ++i) {
dist[i] = 0.0;
}
dist[s] = k;
qt.offer(s);
num[s] = 1;
while (!qt.isEmpty()) {
int x = qt.poll();
in[x] = false;
if (x == s && dist[x] > k) {
return true;
}
for (int t = head[x]; t != -1; t = side[t].next) {
int j = side[t].j;
if (dist[j] < (dist[x] - side[t].c) * side[t].r) {
dist[j] = (dist[x] - side[t].c) * side[t].r;
while (!in[j]) {
++num[j];
if (num[j] >= n) {
return true;
}
in[j] = true;
qt.offer(j);
}
}
}
}
return false;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int n, m, s;
double k;
while (scanner.hasNext()) {
n = scanner.nextInt();
m = scanner.nextInt();
s = scanner.nextInt();
k = scanner.nextDouble();
Arrays.fill(head, -1);
I = 0;
while (m-- > 0) {
int a = scanner.nextInt();
int b = scanner.nextInt();
double rab = scanner.nextDouble();
double cab = scanner.nextDouble();
double rba = scanner.nextDouble();
double cba = scanner.nextDouble();
add(a, b, rab, cab);
add(b, a, rba, cba);
}
if (spfa(s, k, n)) {
System.out.println("YES");
} else {
System.out.println("NO");
}
}
scanner.close();
}
}
```
阅读全文
相关推荐
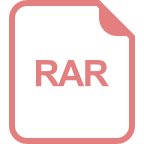
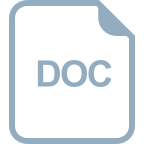
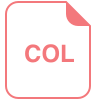
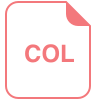
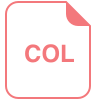








