请用Java编写一个简易五子棋程序可以悔棋,带有界面
时间: 2024-02-13 10:07:38 浏览: 23
好的,我可以为您提供一个简单的五子棋程序,它基于Java Swing构建界面,支持悔棋功能。
代码如下:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class FiveChess extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JButton[][] chessBoard = new JButton[15][15]; // 棋盘网格
private int[][] chessData = new int[15][15]; // 棋盘数据
private JButton regretButton; // 悔棋按钮
private JLabel messageLabel; // 提示信息
private int currentPlayer = 1; // 当前玩家,1表示黑子,2表示白子
private int lastX = -1, lastY = -1; // 上一步棋子的位置
public FiveChess() {
// 初始化窗口
setTitle("五子棋");
setSize(500, 500);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 初始化棋盘
JPanel boardPanel = new JPanel(new GridLayout(15, 15));
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
chessBoard[i][j] = new JButton();
chessBoard[i][j].setBackground(Color.WHITE);
chessBoard[i][j].addActionListener(this);
boardPanel.add(chessBoard[i][j]);
chessData[i][j] = 0;
}
}
// 初始化悔棋按钮和提示信息
regretButton = new JButton("悔棋");
regretButton.addActionListener(this);
messageLabel = new JLabel("黑方先行");
// 将棋盘和按钮添加到窗口
add(boardPanel, BorderLayout.CENTER);
add(regretButton, BorderLayout.NORTH);
add(messageLabel, BorderLayout.SOUTH);
setVisible(true);
}
// 重置棋盘和数据
private void reset() {
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
chessBoard[i][j].setBackground(Color.WHITE);
chessData[i][j] = 0;
}
}
currentPlayer = 1;
lastX = -1;
lastY = -1;
messageLabel.setText("黑方先行");
}
// 判断是否获胜
private boolean isWin(int x, int y) {
int count = 1; // 连续棋子的数量
int color = chessData[x][y]; // 当前玩家的棋子颜色
// 横向判断
for (int i = x - 1; i >= 0 && chessData[i][y] == color; i--) {
count++;
}
for (int i = x + 1; i < 15 && chessData[i][y] == color; i++) {
count++;
}
if (count >= 5) {
return true;
}
// 竖向判断
count = 1;
for (int j = y - 1; j >= 0 && chessData[x][j] == color; j--) {
count++;
}
for (int j = y + 1; j < 15 && chessData[x][j] == color; j++) {
count++;
}
if (count >= 5) {
return true;
}
// 左上-右下斜向判断
count = 1;
for (int i = x - 1, j = y - 1; i >= 0 && j >= 0 && chessData[i][j] == color; i--, j--) {
count++;
}
for (int i = x + 1, j = y + 1; i < 15 && j < 15 && chessData[i][j] == color; i++, j++) {
count++;
}
if (count >= 5) {
return true;
}
// 右上-左下斜向判断
count = 1;
for (int i = x - 1, j = y + 1; i >= 0 && j < 15 && chessData[i][j] == color; i--, j++) {
count++;
}
for (int i = x + 1, j = y - 1; i < 15 && j >= 0 && chessData[i][j] == color; i++, j--) {
count++;
}
if (count >= 5) {
return true;
}
return false;
}
// 下棋
private void playChess(int x, int y) {
// 判断是否已经有棋子
if (chessData[x][y] == 0) {
// 设置棋子颜色
if (currentPlayer == 1) {
chessBoard[x][y].setBackground(Color.BLACK);
chessData[x][y] = 1;
} else {
chessBoard[x][y].setBackground(Color.WHITE);
chessData[x][y] = 2;
}
// 判断是否获胜
if (isWin(x, y)) {
String message = (currentPlayer == 1) ? "黑方获胜!" : "白方获胜!";
JOptionPane.showMessageDialog(this, message);
reset();
} else {
// 切换玩家
currentPlayer = (currentPlayer == 1) ? 2 : 1;
messageLabel.setText((currentPlayer == 1) ? "黑方行动" : "白方行动");
lastX = x;
lastY = y;
}
}
}
// 悔棋
private void regret() {
if (lastX >= 0 && lastY >= 0) {
chessBoard[lastX][lastY].setBackground(Color.WHITE);
chessData[lastX][lastY] = 0;
currentPlayer = (currentPlayer == 1) ? 2 : 1;
messageLabel.setText((currentPlayer == 1) ? "黑方行动" : "白方行动");
}
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == regretButton) {
regret();
} else {
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
if (e.getSource() == chessBoard[i][j]) {
playChess(i, j);
}
}
}
}
}
public static void main(String[] args) {
new FiveChess();
}
}
```
这个五子棋程序使用了Java Swing构建界面,实现了下棋和悔棋功能,支持判断胜负。您可以直接运行这个程序,也可以根据需要进行修改和优化。
相关推荐
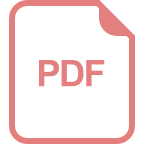














