RSA加密算法优化的java核心代码:DES算法加密明文,RSA加密DES初始密钥
时间: 2023-10-16 07:09:42 浏览: 118
以下是RSA加密算法优化的Java核心代码,包括DES算法加密明文和RSA加密DES初始密钥:
```java
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import java.security.Key;
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.NoSuchAlgorithmException;
public class RSAEncryption {
private static final String RSA_ALGORITHM = "RSA";
private static final String DES_ALGORITHM = "DES";
// 生成DES密钥
public static SecretKey generateDESKey() throws NoSuchAlgorithmException {
KeyGenerator keyGenerator = KeyGenerator.getInstance(DES_ALGORITHM);
keyGenerator.init(56);
return keyGenerator.generateKey();
}
// 使用DES算法加密明文
public static byte[] encrypt(byte[] plainText, Key key) throws Exception {
Cipher cipher = Cipher.getInstance(DES_ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, key);
return cipher.doFinal(plainText);
}
// 使用RSA算法加密DES密钥
public static byte[] encryptKey(Key key, Key publicKey) throws Exception {
Cipher cipher = Cipher.getInstance(RSA_ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
return cipher.doFinal(key.getEncoded());
}
// 使用RSA算法解密DES密钥
public static Key decryptKey(byte[] encryptedKey, Key privateKey) throws Exception {
Cipher cipher = Cipher.getInstance(RSA_ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decryptedKey = cipher.doFinal(encryptedKey);
return new SecretKeySpec(decryptedKey, DES_ALGORITHM);
}
public static void main(String[] args) throws Exception {
// 生成RSA密钥对
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance(RSA_ALGORITHM);
keyPairGenerator.initialize(1024);
KeyPair keyPair = keyPairGenerator.generateKeyPair();
Key publicKey = keyPair.getPublic();
Key privateKey = keyPair.getPrivate();
// 生成DES密钥
SecretKey desKey = generateDESKey();
// 加密明文
String plainText = "Hello world!";
byte[] encryptedPlainText = encrypt(plainText.getBytes(), desKey);
// 加密DES密钥
byte[] encryptedKey = encryptKey(desKey, publicKey);
// 解密DES密钥
Key decryptedKey = decryptKey(encryptedKey, privateKey);
// 解密明文
byte[] decryptedPlainText = encrypt(encryptedPlainText, decryptedKey);
System.out.println("Plain text: " + plainText);
System.out.println("Encrypted plain text: " + new String(encryptedPlainText));
System.out.println("Encrypted key: " + new String(encryptedKey));
System.out.println("Decrypted key: " + new String(decryptedKey.getEncoded()));
System.out.println("Decrypted plain text: " + new String(decryptedPlainText));
}
}
```
在该代码中,我们首先使用`KeyGenerator`生成了一个56位的DES密钥,然后使用`Cipher`类实现了DES算法加密明文和RSA算法加密DES密钥的功能。其中,`Cipher.init()`方法用于初始化加密/解密模式和密钥,`Cipher.doFinal()`方法用于进行加密/解密操作,`Key.getEncoded()`方法用于将密钥编码为字节数组,`SecretKeySpec`类用于将字节数组转换为密钥对象。
最后,我们使用`KeyPairGenerator`生成了RSA密钥对,将公钥用于RSA加密DES密钥,将私钥用于RSA解密DES密钥,从而实现了RSA加密算法优化的Java代码。
阅读全文
相关推荐





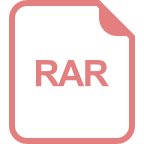


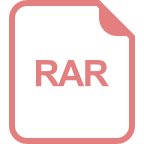

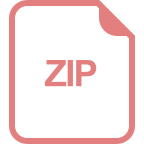







