void printGraphLit( GraphList *G) { /*输出邻接表,输出格式:顶点->邻接顶点编号->...*/ }
时间: 2024-03-09 17:50:43 浏览: 91
以下是输出邻接表的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTEX_NUM 100
// 邻接表节点
typedef struct ArcNode {
int adjvex; // 邻接点的位置
struct ArcNode *next; // 指向下一个邻接点
} ArcNode;
// 顶点信息
typedef struct VertexNode {
int data; // 顶点信息
ArcNode *firstarc; // 指向第一个邻接点
} VertexNode;
// 邻接表
typedef struct GraphList {
VertexNode vertex[MAX_VERTEX_NUM]; // 存放顶点信息
int vexnum; // 顶点数目
int arcnum; // 边数目
} GraphList;
// 输出邻接表
void printGraphList(GraphList *G) {
printf("Adjacency list of the graph:\n");
for (int i = 0; i < G->vexnum; i++) {
printf("%d: ", G->vertex[i].data);
ArcNode *arc = G->vertex[i].firstarc;
while (arc) {
printf("%d ", G->vertex[arc->adjvex].data);
arc = arc->next;
}
printf("\n");
}
}
```
注意事项:
1. 输出格式为 `顶点->邻接顶点编号->...`。
2. 这个函数不需要返回值,直接在函数中输出即可。
阅读全文
相关推荐
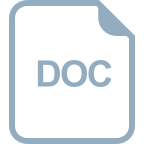
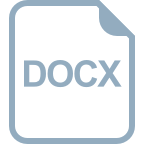
















