python中nn.sequential
时间: 2023-09-26 11:11:22 浏览: 160
`nn.Sequential`是PyTorch中的一个类,用于构建神经网络模型。它可以将多个层按照顺序依次连接起来,形成一个完整的神经网络模型。在实际使用中,可以通过向`nn.Sequential`中传入一个包含各个层的列表或字典来构建模型。
例如,以下代码使用`nn.Sequential`构建了一个简单的全连接神经网络模型:
```
import torch.nn as nn
model = nn.Sequential(
nn.Linear(784, 128),
nn.ReLU(),
nn.Linear(128, 64),
nn.ReLU(),
nn.Linear(64, 10),
nn.LogSoftmax(dim=1)
)
```
在这个例子中,我们构建了一个包含三个全连接层的神经网络模型,其中每个全连接层都是一个`nn.Linear`层,激活函数为ReLU,最后一层为LogSoftmax函数。
使用`nn.Sequential`可以简化模型的构建过程,使代码更加简洁、易于理解。
相关问题
如何改进下面这段代码使其性能更好import torch.nn as nn class fcNN(nn.Module): def __init__(self, in_features): super(fcNN, self).__init__() self.Linear1 = nn.Sequential( nn.Linear(in_features, 8, bias=True), nn.ReLU(), nn.Dropout(0.1) ) self.Linear2 = nn.Sequential( nn.Linear(8, 4, bias=True), nn.ReLU(), nn.Dropout(0.1) ) self.Linear3 = nn.Sequential( nn.Linear(4, 1, bias=True), nn.ReLU(), nn.Dropout(0.1) ) def forward(self, x): x = x.view(1, 1, 1, -1) out = self.Linear3(self.Linear2(self.Linear1(x))) return out
有几种方法可以改进这段代码的性能:
1. 减少 Dropout 层的使用:Dropout 层可以帮助减少过拟合,但是在模型层数较多的情况下使用过多的 Dropout 层会降低模型的性能。可以考虑移除一些 Dropout 层或减小 dropout 概率。
2. 使用更快的激活函数:ReLU 激活函数可以加速模型的训练,但是在一些场景下,如处理稀疏数据时,LeakyReLU 或 ELU 激活函数可能更加适合。
3. 减少层数:如果模型层数过多,可以考虑减少层数或减小每层的神经元数量。
下面是一个修改后的代码示例:
```python
import torch.nn as nn
class fcNN(nn.Module):
def __init__(self, in_features):
super(fcNN, self).__init__()
self.Linear1 = nn.Sequential(
nn.Linear(in_features, 8, bias=True),
nn.LeakyReLU()
)
self.Linear2 = nn.Sequential(
nn.Linear(8, 4, bias=True),
nn.LeakyReLU()
)
self.Linear3 = nn.Linear(4, 1, bias=True)
def forward(self, x):
x = x.view(1, 1, 1, -1)
out = self.Linear3(self.Linear2(self.Linear1(x)))
return out
```
这个修改后的代码移除了 Dropout 层,并使用了更快的 LeakyReLU 激活函数。另外,模型的层数也减少为三层,每层的神经元数量也减少为了 8 和 4。这些修改都可以提高模型的性能。
nn.ModuleList和nn.Sequential
在PyTorch中,`nn.ModuleList` 和 `nn.Sequential` 都是神经网络模块(Modules)的容器,但它们的设计目的和使用场景有所不同。
`nn.ModuleList`是一个动态大小的模块列表,它可以存储一系列独立的模块,并通过索引来访问和遍历这些模块。每当添加或删除模块时,这个列表都会自动调整其内部结构。你可以在训练过程中动态地向 `ModuleList` 添加或移除层,这在构建可变长度的模型结构时特别有用。
例如:
```python
module_list = nn.ModuleList([nn.Linear(10, 5), nn.ReLU()])
```
在这个例子中,`module_list` 包含了两个线性和一个ReLU激活函数。
相比之下,`nn.Sequential`是线性的顺序模块列表,它的模块按照添加的顺序执行。每个模块的输出直接成为下一个模块的输入。它适合构建固定的、有明确执行顺序的网络架构,如前馈神经网络。
例如:
```python
sequential = nn.Sequential(nn.Linear(10, 5), nn.ReLU())
```
这里,`sequential` 就会先执行线性变换,再接上ReLU激活。
阅读全文
相关推荐
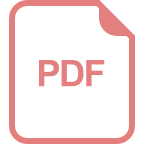














