self.encoder = nn.Sequential(nn.Linear(65, 32, bias=False), nn.BatchNorm1d(32), nn.ReLU(inplace=True), # first layer nn.Linear(32, 32, bias=False), nn.BatchNorm1d(32), nn.ReLU(inplace=True), # second layer nn.Linear(32, 65, bias=False), nn.BatchNorm1d(65, affine=False)) # output layer # build a 2-layer predictor self.predictor = nn.Sequential(nn.Linear(65, 32, bias=False), nn.BatchNorm1d(32), nn.ReLU(inplace=True), # hidden layer nn.Linear(32, 65)) # output layer 我的网络设计成这样请帮我写一个测试的代码
时间: 2024-02-10 21:10:12 浏览: 242
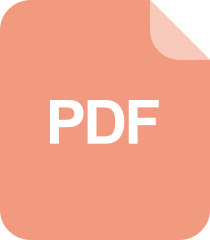
pytorch 中的重要模块化接口nn.Module的使用
好的,下面是一个简单的测试代码,你可以根据自己的需求进行修改:
```python
import torch
from torch import nn
# 构建模型
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.encoder = nn.Sequential(
nn.Linear(65, 32, bias=False),
nn.BatchNorm1d(32),
nn.ReLU(inplace=True),
nn.Linear(32, 32, bias=False),
nn.BatchNorm1d(32),
nn.ReLU(inplace=True),
nn.Linear(32, 65, bias=False),
nn.BatchNorm1d(65, affine=False)
)
self.predictor = nn.Sequential(
nn.Linear(65, 32, bias=False),
nn.BatchNorm1d(32),
nn.ReLU(inplace=True),
nn.Linear(32, 65)
)
def forward(self, x):
x = self.encoder(x)
x = self.predictor(x)
return x
# 测试模型
model = MyModel()
input_data = torch.randn(10, 65) # 生成10个样本,每个样本包含65个特征
output = model(input_data)
print(output.shape) # 输出为(10, 65),即10个样本,每个样本有65个特征
```
注意:这里的测试代码仅为示例,实际应用中还需要根据任务需要进行修改和调整。
阅读全文
相关推荐
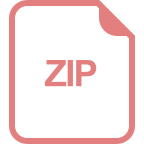
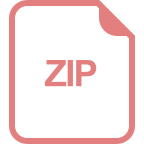
















