std::cout和std::endl函数
时间: 2023-05-29 15:03:57 浏览: 279
std::cout是C++中的一个输出流对象,用于将数据输出到控制台或其他输出设备。它是iostream库中的一部分,可以使用<<运算符将数据插入到输出流中。
std::endl是一个输出控制符,用于在输出流中插入一个换行符并刷新缓冲区。它等价于使用'\n'插入一个换行符并调用std::flush函数刷新输出流缓冲区。
使用std::cout和std::endl可以方便地输出数据到控制台或其他输出设备,并保证输出的正确性和可读性。例如:
std::cout << "Hello, World!" << std::endl;
将输出"Hello, World!"并在末尾插入一个换行符。
相关问题
std::cin和std::cout和std::endl
std::cin和std::cout都是C++标准库中的流对象,分别用于从标准输入流中读取数据和向标准输出流中写入数据。两者都需要包含头文件iostream来使用。
std::endl是C++标准库中的一个操作符,用于向输出流中插入一个换行符,并刷新输出缓冲区。通常情况下,我们可以使用'\n'来表示换行符,但使用std::endl可以保证在输出换行符后立即刷新缓冲区,确保输出的数据可以及时显示在屏幕上。例如,可以使用std::cout << "Hello world!" << std::endl;输出"Hello world!"并换行。
需要注意的是,std::endl是一个操作符,而不是一个普通的函数或变量,因此不需要使用括号来调用。
std std::cout
std是C++标准库的命名空间,它包含了许多常用的类和函数。其中,std::cout是std命名空间中的一个对象,用于向标准输出流(通常是控制台)输出数据。
在C++中,我们可以使用std::cout来输出文本、变量值等信息。例如,下面的代码将输出"Hello, World!"到控制台:
```cpp
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
```
在这个例子中,`<<`是一个输出运算符,它将字符串"Hello, World!"插入到std::cout对象中,并通过`std::endl`来结束一行并刷新输出流。
需要注意的是,在使用std::cout之前,我们需要包含头文件<iostream>。这个头文件定义了std::cout和其他与输入输出相关的类和函数。
希望这个回答能够帮助你理解std和std::cout的概念。如果你还有其他问题,请随时提问。
相关推荐
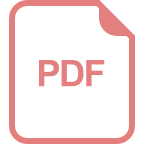
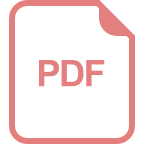
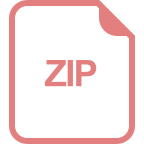
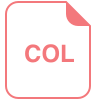
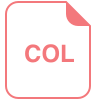










