用c++写出代码 括号匹配
时间: 2023-05-28 12:07:48 浏览: 67
以下是用C语言实现括号匹配的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STACK_SIZE 100
typedef struct {
char data[MAX_STACK_SIZE];
int top;
} Stack;
void init(Stack *s) {
s->top = -1;
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_full(Stack *s) {
return s->top == MAX_STACK_SIZE - 1;
}
void push(Stack *s, char c) {
if (is_full(s)) {
printf("Stack overflow\n");
exit(EXIT_FAILURE);
}
s->data[++s->top] = c;
}
char pop(Stack *s) {
if (is_empty(s)) {
printf("Stack underflow\n");
exit(EXIT_FAILURE);
}
return s->data[s->top--];
}
int is_matching_pair(char left, char right) {
if (left == '(' && right == ')') {
return 1;
} else if (left == '{' && right == '}') {
return 1;
} else if (left == '[' && right == ']') {
return 1;
}
return 0;
}
int is_balanced(char *exp) {
Stack s;
init(&s);
for (int i = 0; i < strlen(exp); i++) {
if (exp[i] == '(' || exp[i] == '{' || exp[i] == '[') {
push(&s, exp[i]);
} else if (exp[i] == ')' || exp[i] == '}' || exp[i] == ']') {
if (is_empty(&s) || !is_matching_pair(pop(&s), exp[i])) {
return 0;
}
}
}
return is_empty(&s);
}
int main() {
char exp[MAX_STACK_SIZE];
printf("Enter an expression: ");
fgets(exp, MAX_STACK_SIZE, stdin);
exp[strcspn(exp, "\n")] = '\0'; // remove trailing newline from fgets
if (is_balanced(exp)) {
printf("The expression is balanced\n");
} else {
printf("The expression is not balanced\n");
}
return 0;
}
```
运行示例:
```
Enter an expression: (){}[]
The expression is balanced
```
```
Enter an expression: ((()))
The expression is balanced
```
```
Enter an expression: ({)}
The expression is not balanced
```
阅读全文
相关推荐

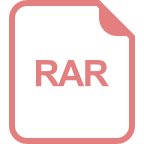















