tensorflow中voc2007数据集转换成Tfrecord文件
时间: 2023-09-18 14:15:51 浏览: 143
将VOC2007数据集转换为TFRecord文件需要以下步骤:
1. 下载VOC2007数据集并解压缩。
2. 安装TensorFlow和Pillow库。
3. 编写脚本将VOC2007数据集转换为TFRecord文件。
以下是一个简单的Python脚本示例,可以将VOC2007数据集转换为TFRecord文件:
```python
import tensorflow as tf
import os
import io
import xml.etree.ElementTree as ET
from PIL import Image
def create_tf_example(example):
# 读取图像文件
img_path = os.path.join('VOCdevkit/VOC2007/JPEGImages', example['filename'])
with tf.io.gfile.GFile(img_path, 'rb') as fid:
encoded_jpg = fid.read()
encoded_jpg_io = io.BytesIO(encoded_jpg)
image = Image.open(encoded_jpg_io)
width, height = image.size
# 读取标注文件
xml_path = os.path.join('VOCdevkit/VOC2007/Annotations', example['filename'].replace('.jpg', '.xml'))
with tf.io.gfile.GFile(xml_path, 'r') as fid:
xml_str = fid.read()
xml = ET.fromstring(xml_str)
# 解析标注文件
xmins = []
xmaxs = []
ymins = []
ymaxs = []
classes_text = []
classes = []
for obj in xml.findall('object'):
class_name = obj.find('name').text
classes_text.append(class_name.encode('utf8'))
classes.append(label_map[class_name])
bbox = obj.find('bndbox')
xmins.append(float(bbox.find('xmin').text) / width)
ymins.append(float(bbox.find('ymin').text) / height)
xmaxs.append(float(bbox.find('xmax').text) / width)
ymaxs.append(float(bbox.find('ymax').text) / height)
# 构造TFRecord Example
tf_example = tf.train.Example(features=tf.train.Features(feature={
'image/height': tf.train.Feature(int64_list=tf.train.Int64List(value=[height])),
'image/width': tf.train.Feature(int64_list=tf.train.Int64List(value=[width])),
'image/filename': tf.train.Feature(bytes_list=tf.train.BytesList(value=[example['filename'].encode('utf8')])),
'image/source_id': tf.train.Feature(bytes_list=tf.train.BytesList(value=[example['filename'].encode('utf8')])),
'image/encoded': tf.train.Feature(bytes_list=tf.train.BytesList(value=[encoded_jpg])),
'image/format': tf.train.Feature(bytes_list=tf.train.BytesList(value=['jpeg'.encode('utf8')])),
'image/object/bbox/xmin': tf.train.Feature(float_list=tf.train.FloatList(value=xmins)),
'image/object/bbox/xmax': tf.train.Feature(float_list=tf.train.FloatList(value=xmaxs)),
'image/object/bbox/ymin': tf.train.Feature(float_list=tf.train.FloatList(value=ymins)),
'image/object/bbox/ymax': tf.train.Feature(float_list=tf.train.FloatList(value=ymaxs)),
'image/object/class/text': tf.train.Feature(bytes_list=tf.train.BytesList(value=classes_text)),
'image/object/class/label': tf.train.Feature(int64_list=tf.train.Int64List(value=classes)),
}))
return tf_example
# 将VOC2007数据集转换为TFRecord文件
def create_tf_record(output_file):
examples = [...] # 从VOC2007数据集读取实例
writer = tf.io.TFRecordWriter(output_file)
for example in examples:
tf_example = create_tf_example(example)
writer.write(tf_example.SerializeToString())
writer.close()
label_map = {...} # 标签映射
output_file = 'voc2007_train.tfrecord'
create_tf_record(output_file)
```
其中`create_tf_example`函数将一个VOC2007样本转换为TFRecord Example,`create_tf_record`函数将整个VOC2007数据集转换为TFRecord文件。在这个例子中,我们假设VOC2007数据集已经被解压缩到`VOCdevkit/VOC2007`目录下,标签映射已经定义为`label_map`变量。你需要根据自己的实际情况修改这些变量。
阅读全文
相关推荐
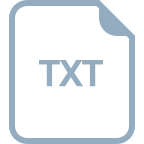
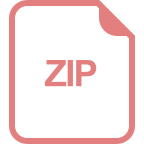

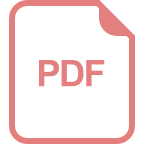
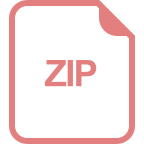
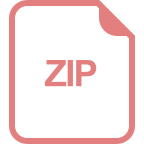
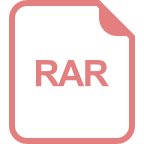
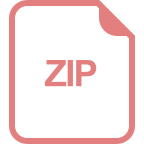
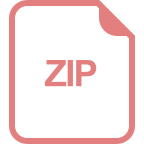
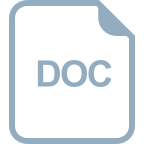
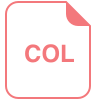
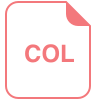
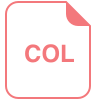
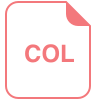
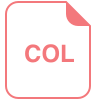
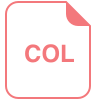
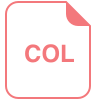
