opencv python如何拟合直线
时间: 2023-09-10 21:11:00 浏览: 66
你可以使用OpenCV的函数cv2.fitLine()来拟合直线。具体的代码实现如下:
```python
import cv2
import numpy as np
# 生成一些随机的点
points = np.random.randint(0, 100, size=(50,2))
# 使用cv2.fitLine()函数拟合直线
vx, vy, cx, cy = cv2.fitLine(points, cv2.DIST_L2, 0, 0.01, 0.01)
# 计算直线的斜率和截距
k = vy / vx
b = cy - k * cx
# 打印直线的斜率和截距
print("斜率: ", k)
print("截距: ", b)
```
在上面的代码中,我们首先生成了一些随机的点,并且使用cv2.fitLine()函数拟合出了一条直线。然后,我们计算了直线的斜率和截距,并将它们打印出来。
相关问题
python opencv轮廓拟合直线
要在Python中使用OpenCV进行轮廓拟合直线,可以使用cv2.fitLine()函数。该函数的定义如下: def fitLine(points, distType, param, reps, aeps, line=None)。 = cv2.fitLine(contours<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [轮廓拟合---OpenCV-Python开发指南(27)](https://blog.csdn.net/liyuanjinglyj/article/details/113921491)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
OpenCV Python如何拟合直线
可以使用OpenCV库的Hough变换函数来拟合直线。具体步骤是首先进行边缘检测,然后调用HoughLines函数检测直线,最后使用polylines函数绘制检测到的直线。代码示例:
import cv2
import numpy as np
# 读入图像
img = cv2.imread('input_image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 边缘检测
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
# Hough变换检测直线
lines = cv2.HoughLines(edges, 1, np.pi/180, 200)
# 绘制检测到的直线
for line in lines:
rho, theta = line[0]
a = np.cos(theta)
b = np.sin(theta)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000*(-b))
y1 = int(y0 + 1000*(a))
x2 = int(x0 - 1000*(-b))
y2 = int(y0 - 1000*(a))
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
# 显示结果
cv2.imshow('result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
相关推荐
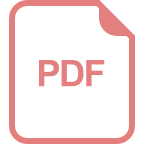












