用matlab实现牛顿迭代法
时间: 2023-05-20 18:00:41 浏览: 227
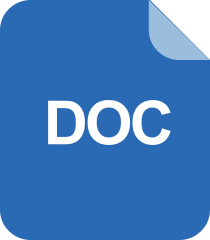
牛顿迭代法的matlab编程

可以使用以下代码实现牛顿迭代法:
function [x, iter] = newton(f, df, x0, tol, maxiter)
% f: 目标函数
% df: 目标函数的导数
% x0: 初始值
% tol: 容差
% maxiter: 最大迭代次数
iter = 0;
x = x0;
while abs(f(x)) > tol && iter < maxiter
x = x - f(x) / df(x);
iter = iter + 1;
end
if iter == maxiter
disp('达到最大迭代次数,未能收敛');
end
end
其中,f和df分别为目标函数和目标函数的导数,x0为初始值,tol为容差,maxiter为最大迭代次数。
阅读全文
相关推荐
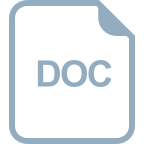
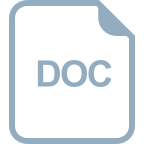


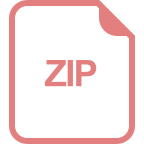
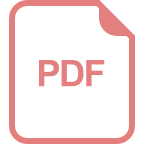
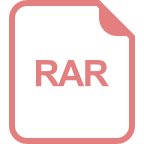
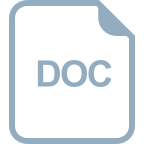
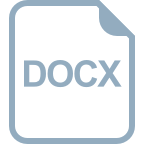
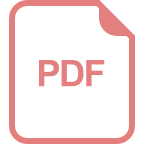
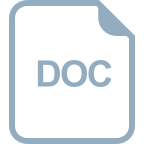
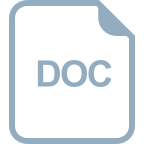
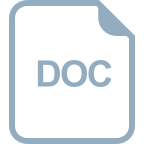
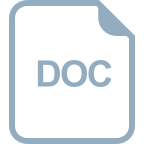

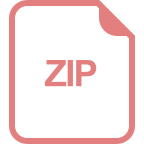
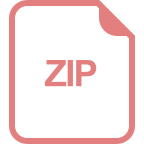
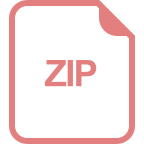