python控制arduino_Python课程系列:使用python将PC与arduino板连接控制LED
时间: 2024-02-22 15:54:48 浏览: 156
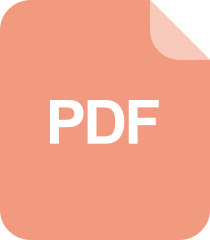
谈如何用Python控制Arduino.pdf

好的,我可以回答这个问题。首先,你需要确保你的电脑已经安装了Arduino IDE和Python。接下来,你需要连接你的Arduino板到电脑上,然后打开Arduino IDE,选择示例代码中的“Blink”程序并上传到Arduino板上。
然后,你可以打开Python并安装pyserial库。使用以下代码打开串口连接:
```python
import serial
ser = serial.Serial('COM3', 9600) #根据你的Arduino板连接的串口号和波特率进行更改
```
接下来,你可以使用以下代码来控制LED灯:
```python
ser.write(b'H') #发送字符'H'到Arduino板
```
Arduino板会接收到'H'字符并执行相应的操作,例如控制LED灯的开和关。你可以使用以下代码来关闭LED灯:
```python
ser.write(b'L') #发送字符'L'到Arduino板
```
最后,当你完成了你的操作后,别忘了关闭串口连接:
```python
ser.close() #关闭串口连接
```
希望这些代码能帮助你控制Arduino板上的LED灯。
阅读全文
相关推荐
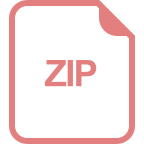
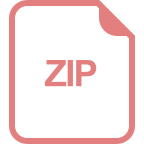
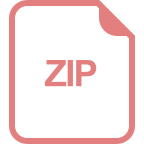
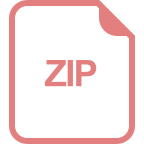
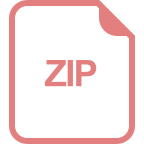
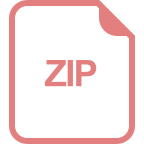
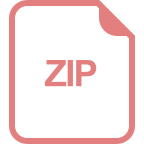
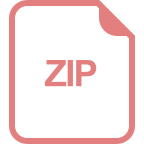
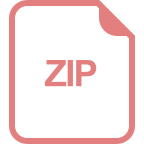
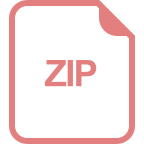
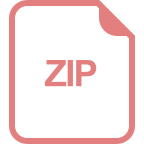
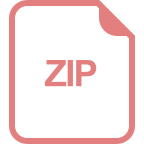
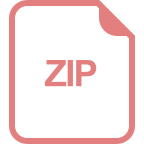
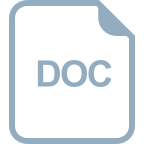


