使用jieba分词器,对raw_title列每一个商品标题进行分词,通过停用表StopWords对标题进行去除停用词。因为下面要统计每个词语的个数,所以 为了准确性,在这里对过滤后的数据 title_clean 中的每个list的元素进行去重,即每个标题被分割后的词语唯一
时间: 2024-04-09 17:33:03 浏览: 27
要使用 jieba 分词器对 "raw_title" 列的每个商品标题进行分词,并通过停用词表进行停用词处理,并且在过滤后的数据中对每个列表元素进行去重,可以按照以下步骤进行操作:
首先,导入所需的库和停用词表:
```python
import jieba
from collections import Counter
# 停用词表路径
stopwords_path = "stopwords.txt"
```
接下来,定义一个函数来对标题进行分词和去除停用词的处理:
```python
def process_title(title):
# 分词
words = jieba.cut(title)
# 去除停用词
words = [word for word in words if word not in stopwords]
# 去重
words = list(set(words))
return words
```
然后,读取数据并加载停用词表:
```python
# 读取数据
data = pd.read_csv("data.csv")
# 加载停用词表
with open(stopwords_path, "r", encoding="utf-8") as f:
stopwords = [line.strip() for line in f.readlines()]
```
最后,应用函数 `process_title` 对 "raw_title" 列进行处理,并统计每个词语的个数:
```python
# 对标题进行分词和去除停用词的处理,并统计每个词语的个数
data["title_clean"] = data["raw_title"].apply(process_title)
word_counts = Counter([word for words in data["title_clean"] for word in words])
```
上述代码将在 DataFrame 中创建一个名为 "title_clean" 的新列,其中包含处理后的标题数据。然后,使用 `Counter` 对所有标题的词语进行计数。
请注意,您需要将停用词表的路径 `stopwords_path` 替换为您自己的停用词表路径。
希望以上解释能够帮助您实现所需的功能。如果您有任何其他问题,请随时提问。
相关推荐
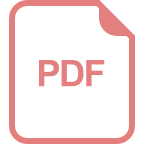
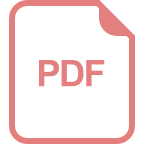
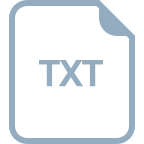
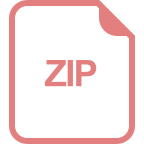
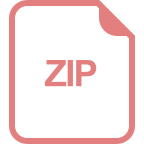
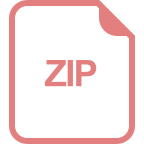
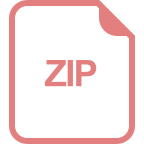
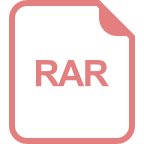
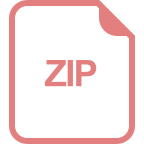
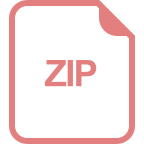
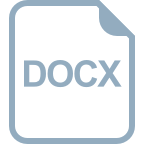
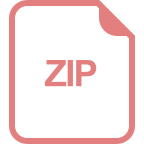