使用FileInputStream、FileOutputStreaem和FileReader、FileWriter分别进行拷贝。 (2)使用字节流拷贝时, 定义一个1024长度的字节数组作为缓冲区,使用字符流拷贝。
时间: 2024-02-29 20:51:37 浏览: 72
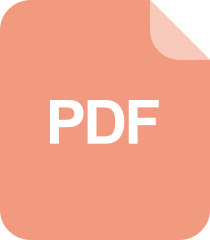
IO流文件操作字节流使用
好的,我明白了你的问题。以下是对你的问题的回答:
1. 使用FileInputStream、FileOutputStreaem和FileReader、FileWriter分别进行拷贝:
使用FileInputStream和FileOutputStream进行拷贝:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
try {
FileInputStream in = new FileInputStream("source.txt");
FileOutputStream out = new FileOutputStream("target.txt");
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
in.close();
out.close();
System.out.println("File copied successfully!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
使用FileReader和FileWriter进行拷贝:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
try {
FileReader in = new FileReader("source.txt");
FileWriter out = new FileWriter("target.txt");
int c;
while ((c = in.read()) != -1) {
out.write(c);
}
in.close();
out.close();
System.out.println("File copied successfully!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
2. 使用字节流拷贝时, 定义一个1024长度的字节数组作为缓冲区,使用字符流拷贝。
使用字节流拷贝:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
try {
FileInputStream in = new FileInputStream("source.txt");
FileOutputStream out = new FileOutputStream("target.txt");
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
in.close();
out.close();
System.out.println("File copied successfully!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
使用字符流拷贝:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
try {
FileReader in = new FileReader("source.txt");
FileWriter out = new FileWriter("target.txt");
char[] buffer = new char[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
in.close();
out.close();
System.out.println("File copied successfully!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
希望这些代码可以帮助到你!
阅读全文
相关推荐
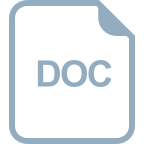
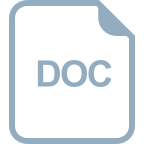














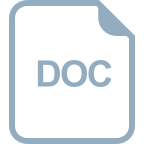
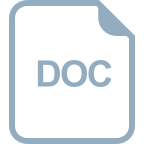
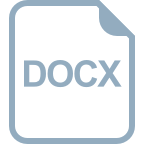