matlab隐含波动率函数
时间: 2023-08-06 14:17:27 浏览: 49
Matlab中可以使用“blsimpv”函数来计算隐含波动率。这个函数需要输入期权价格、标的资产价格、执行价格、到期时间、无风险利率和期权类型等参数。下面是一个简单的示例代码:
```matlab
S = 100; % 标的资产价格
K = 100; % 执行价格
r = 0.05; % 无风险利率
T = 1; % 到期时间
sigma = 0.2; % 初始猜测波动率
CallPrice = 10; % 期权价格
PutPrice = 5;
OptSpec = 'call'; % 期权类型
% 使用fzero函数求解隐含波动率
IV = fzero(@(x) (blsprice(S, K, r, T, x) - CallPrice), sigma);
% 使用blsimpv函数求解隐含波动率
IV2 = blsimpv(S, K, r, T, CallPrice, [], [], [], OptSpec);
% 输出结果
disp(['使用fzero函数求解的隐含波动率为:' num2str(IV)]);
disp(['使用blsimpv函数求解的隐含波动率为:' num2str(IV2)]);
```
这段代码首先定义了一些参数,然后使用“fzero”函数和“blsimpv”函数分别计算了隐含波动率。其中,“fzero”函数使用了Black-Scholes公式计算期权价格,而“blsimpv”函数直接计算出了隐含波动率。最后,将两种方法计算出的隐含波动率输出到屏幕上。
相关问题
matlab计算期权隐含波动率的代码
计算期权隐含波动率的代码可以用MATLAB的Financial Toolbox中的blsimpv函数。blsimpv函数是根据Black-Scholes模型计算期权的隐含波动率的。
以下是一个示例代码:
```matlab
% 假设期权信息如下:
S = 100; % 标的资产价格
K = 100; % 行权价格
r = 0.05; % 无风险利率
T = 1; % 到期时间
MarketPrice = 10; % 期权市场价格
% 使用blsimpv函数计算隐含波动率
iv = blsimpv(S, K, r, T, MarketPrice);
% 显示计算结果
fprintf('隐含波动率为 %.4f\n', iv);
```
blsimpv函数的参数含义如下:
- S:标的资产价格
- K:行权价格
- r:无风险利率
- T:到期时间
- MarketPrice:期权市场价格
- iv:隐含波动率
matlab绘图波动率微笑
在金融领域,波动率微笑是指在同一时间到期的不同执行价格的期权的隐含波动率呈现出一种“微笑状”的曲线。在Matlab中,我们可以使用“blsprice”函数和“blsimpv”函数来计算期权价格和隐含波动率,然后使用“plot”函数来绘制波动率微笑曲线。下面是一个示例代码:
```matlab
S = 100; % 标的资产价格
r = 0.05; % 无风险利率
T = 1; % 到期时间
K = [80:120]; % 执行价格范围
sigma = 0.2; % 初始猜测波动率
OptSpec = 'call'; % 期权类型
% 使用blsimpv函数计算不同执行价格的隐含波动率
IV = blsimpv(S, K, r, T, [], [], [], [], OptSpec);
% 绘制波动率微笑曲线
plot(K, IV, 'b-', 'LineWidth', 1.5);
xlabel('执行价格');
ylabel('隐含波动率');
title('波动率微笑曲线');
```
这段代码使用“blsimpv”函数计算了不同执行价格的期权的隐含波动率,并将结果存储在变量“IV”中。然后,使用“plot”函数绘制了波动率微笑曲线,其中横坐标为执行价格,纵坐标为隐含波动率。最后,使用“xlabel”、“ylabel”和“title”函数添加了横轴、纵轴和标题。你可以根据需要修改代码中的参数来绘制不同形状的波动率微笑曲线。
相关推荐
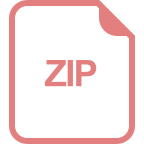
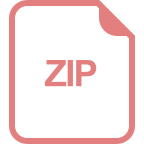
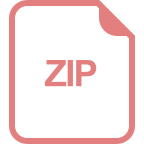







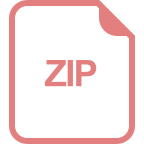
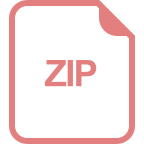
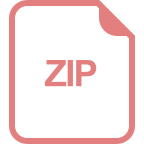
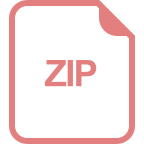
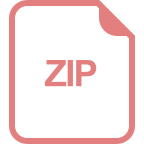
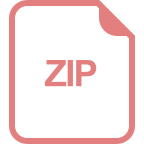