编写一个程序,可以在命令行输入参数,完成指定文件的直方图均衡,并存储到新文件,命令行参数如下 hist file1.bmp file2.bmp 第一个参数为可执行程序名称,第二个参数为原始图像文件名,第三个参数为新文件名(c语言代码)
时间: 2024-02-15 12:06:09 浏览: 80
以下是一个简单的 C 语言程序,可以实现指定文件的直方图均衡并存储到新文件:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BMP_HEADER_SIZE 54
#define HISTOGRAM_SIZE 256
int main(int argc, char *argv[])
{
FILE *in_file, *out_file;
char *in_file_name, *out_file_name;
unsigned char header[BMP_HEADER_SIZE];
unsigned char *image_data;
int image_size, width, height, i, j;
int histogram[HISTOGRAM_SIZE] = {0};
float cdf[HISTOGRAM_SIZE] = {0};
int new_histogram[HISTOGRAM_SIZE] = {0};
int new_pixel_value;
// 检查参数个数
if (argc != 3) {
printf("Usage: hist <input_file> <output_file>\n");
return 1;
}
// 获取文件名
in_file_name = argv[1];
out_file_name = argv[2];
// 打开输入文件
in_file = fopen(in_file_name, "rb");
if (in_file == NULL) {
printf("Error: cannot open input file %s\n", in_file_name);
return 1;
}
// 读取 BMP 文件头
fread(header, sizeof(unsigned char), BMP_HEADER_SIZE, in_file);
// 获取图像大小和宽高
image_size = *(int*)(header+2);
width = *(int*)(header+18);
height = *(int*)(header+22);
// 分配内存并读取图像数据
image_data = (unsigned char*) malloc(image_size);
fread(image_data, sizeof(unsigned char), image_size, in_file);
// 关闭输入文件
fclose(in_file);
// 计算直方图
for (i = 0; i < image_size; i++) {
histogram[image_data[i]]++;
}
// 计算累积分布函数
cdf[0] = (float) histogram[0] / (float) (width * height);
for (i = 1; i < HISTOGRAM_SIZE; i++) {
cdf[i] = cdf[i-1] + (float) histogram[i] / (float) (width * height);
}
// 映射像素值
for (i = 0; i < HISTOGRAM_SIZE; i++) {
new_pixel_value = (int) (cdf[i] * (float) (HISTOGRAM_SIZE - 1) + 0.5);
new_histogram[new_pixel_value] += histogram[i];
}
// 修改 BMP 文件头中像素数据大小
*(int*)(header+2) = width * height * sizeof(unsigned char);
// 打开输出文件
out_file = fopen(out_file_name, "wb");
if (out_file == NULL) {
printf("Error: cannot open output file %s\n", out_file_name);
return 1;
}
// 写入 BMP 文件头和修改后的图像数据
fwrite(header, sizeof(unsigned char), BMP_HEADER_SIZE, out_file);
fwrite(new_histogram, sizeof(unsigned char), width * height, out_file);
// 关闭输出文件
fclose(out_file);
// 释放内存
free(image_data);
return 0;
}
```
该程序使用了一个长度为 256 的数组来表示图像的直方图,然后计算出累积分布函数,并根据累积分布函数来映射像素值,最后将修改后的图像数据和原始 BMP 文件头一起写入到新文件中。注意,该程序仅支持 24 位 BMP 图像。
阅读全文
相关推荐
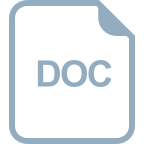
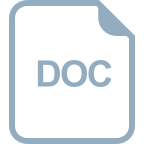
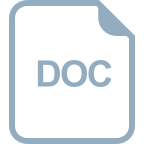




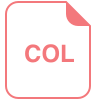
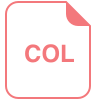
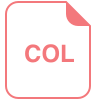
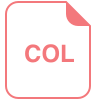
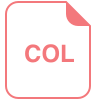
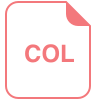
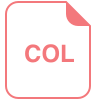
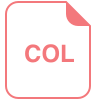
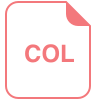
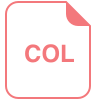
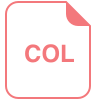
