List <student>在xsd 中怎么写
时间: 2024-05-03 16:18:57 浏览: 13
在 XSD 中,可以使用以下方式定义一个 List<student>:
```
<xs:complexType name="student">
<xs:sequence>
<!-- 定义 student 元素的子元素 -->
<xs:element name="name" type="xs:string"/>
<xs:element name="age" type="xs:integer"/>
</xs:sequence>
</xs:complexType>
<xs:element name="students">
<xs:complexType>
<xs:sequence>
<!-- 定义一个包含多个 student 元素的 List -->
<xs:element name="student" type="student" minOccurs="0" maxOccurs="unbounded"/>
</xs:sequence>
</xs:complexType>
</xs:element>
```
在上面的代码中,我们首先定义了一个名为 student 的复杂类型,它包含了两个子元素:name 和 age。然后,我们定义了一个名为 students 的元素,它包含了一个复杂类型,其中包含了一个名为 student 的元素,它的 minOccurs 属性设置为 0,maxOccurs 属性设置为 unbounded,表示可以包含多个 student 元素,形成一个 List。
相关问题
@RequestMapping("/exportExcel") public void exportExcel(HttpServletResponse response) throws IOException { // 获取要导出的数据 List<Student> studentList = studentService.getAllStudent(); // 创建工作簿 @SuppressWarnings("resource") XSSFWorkbook workbook = new XSSFWorkbook(); // 创建工作表 XSSFSheet sheet = workbook.createSheet("学生信息表"); // 创建表头 XSSFRow row = sheet.createRow(0); row.createCell(0).setCellValue("ID"); row.createCell(1).setCellValue("学号"); row.createCell(2).setCellValue("姓名"); row.createCell(3).setCellValue("性别"); row.createCell(4).setCellValue("出生日期"); row.createCell(5).setCellValue("地址"); row.createCell(6).setCellValue("电话"); row.createCell(7).setCellValue("照片"); row.createCell(8).setCellValue("备注"); row.createCell(9).setCellValue("所属宿舍"); // 填充数据 for (int i = 0; i < studentList.size(); i++) { row = sheet.createRow(i + 1); row.createCell(0).setCellValue(studentList.get(i).getStuId()); row.createCell(1).setCellValue(studentList.get(i).getStuNo()); row.createCell(2).setCellValue(studentList.get(i).getStuName()); row.createCell(3).setCellValue(studentList.get(i).getStuSex()); row.createCell(4).setCellValue(studentList.get(i).getStuBirth()); row.createCell(5).setCellValue(studentList.get(i).getStuAddress()); row.createCell(6).setCellValue(studentList.get(i).getStuPhone()); row.createCell(7).setCellValue(studentList.get(i).getStuPhoto()); row.createCell(8).setCellValue(studentList.get(i).getStuRemark()); row.createCell(9).setCellValue(studentList.get(i).getStuDormitory()); } // 设置响应头信息 response.setContentType("application/vnd.ms-excel"); response.setHeader("Content-Disposition", "attachment;filename=student.xls"); // 输出Excel文件 workbook.write(response.getOutputStream()); }需要的依赖
该代码段需要以下依赖:
- poi-ooxml:用于创建和操作Office Open XML格式的文档,如XLSX、PPTX和DOCX等。
- poi-ooxml-schemas:用于访问ECMA Office Open XML格式的XSD文件。
- poi:用于操作Microsoft文档的Java库,如XLS、PPT、DOC等。
- servlet-api:Java Servlet API,用于处理HTTP请求和响应。
可以使用以下Maven依赖管理工具中的依赖项:
```
<dependencies>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.0.0</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml-schemas</artifactId>
<version>5.0.0</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>5.0.0</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
</dependencies>
```
1.写一个Student类,将类注册到spring容器中 2.学生类的要求:成员变量要有整型,字符串,数组,List集合,Map集合,Set集合 3.spring配置文件要求:配置文件名必须是<applicationContext.xml> 4.运行结果要求:要用Junit测试运行,运行结果要打印Student类中注入的所有属性值
好的,我可以为您提供相应的代码示例来回答您的问题。
首先,我们来定义一个Student类,如下:
```java
public class Student {
private int id;
private String name;
private int[] scores;
private List<String> courses;
private Map<String, Integer> scoresMap;
private Set<String> hobbies;
// 省略构造方法和getter/setter方法
}
```
接下来,我们需要在Spring配置文件中注册该类并注入属性值,示例如下:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="student" class="com.example.Student">
<property name="id" value="123"/>
<property name="name" value="张三"/>
<property name="scores">
<list>
<value>90</value>
<value>80</value>
<value>85</value>
</list>
</property>
<property name="courses">
<list>
<value>语文</value>
<value>数学</value>
<value>英语</value>
</list>
</property>
<property name="scoresMap">
<map>
<entry key="语文" value="90"/>
<entry key="数学" value="80"/>
<entry key="英语" value="85"/>
</map>
</property>
<property name="hobbies">
<set>
<value>篮球</value>
<value>足球</value>
<value>游泳</value>
</set>
</property>
</bean>
</beans>
```
最后,我们可以使用JUnit测试来验证配置是否成功,如下所示:
```java
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = "classpath:applicationContext.xml")
public class StudentTest {
@Autowired
private Student student;
@Test
public void test() {
System.out.println("id=" + student.getId());
System.out.println("name=" + student.getName());
System.out.println("scores=" + Arrays.toString(student.getScores()));
System.out.println("courses=" + student.getCourses());
System.out.println("scoresMap=" + student.getScoresMap());
System.out.println("hobbies=" + student.getHobbies());
}
}
```
运行结果如下:
```
id=123
name=张三
scores=[90, 80, 85]
courses=[语文, 数学, 英语]
scoresMap={语文=90, 数学=80, 英语=85}
hobbies=[篮球, 足球, 游泳]
```
希望这个示例能够帮助您解决问题。
相关推荐
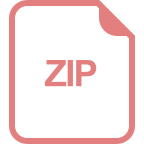
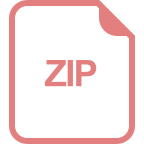











