generation_config.max_length = generation_config.max_new_tokens + input_ids_seq_length if not has_default_max_length: logger.warn( f"Both `max_new_tokens` (={generation_config.max_new_tokens}) and `max_length`(=" f"{generation_config.max_length}) seem to have been set. `max_new_tokens` will take precedence. " "Please refer to the documentation for more information. " "(https://huggingface.co/docs/transformers/main/en/main_classes/text_generation)", UserWarning, )
时间: 2024-04-27 17:24:31 浏览: 163
这是来自于Hugging Face Transformers库中的一段代码,它会根据输入序列的长度(`input_ids_seq_length`)以及`max_new_tokens`参数的值来计算生成文本的最大长度(`max_length`)。如果同时设置了`max_new_tokens`和`max_length`,则`max_new_tokens`的值将被优先使用。如果你在使用Hugging Face Transformers进行文本生成时遇到了相关的警告信息,可以参考文档中提供的信息进行解决。
相关问题
import tensorflow as tf import tensorflow_hub as hub from tensorflow.keras import layers import bert import numpy as np from transformers import BertTokenizer, BertModel # 设置BERT模型的路径和参数 bert_path = "E:\\AAA\\523\\BERT-pytorch-master\\bert1.ckpt" max_seq_length = 128 train_batch_size = 32 learning_rate = 2e-5 num_train_epochs = 3 # 加载BERT模型 def create_model(): input_word_ids = tf.keras.layers.Input(shape=(max_seq_length,), dtype=tf.int32, name="input_word_ids") input_mask = tf.keras.layers.Input(shape=(max_seq_length,), dtype=tf.int32, name="input_mask") segment_ids = tf.keras.layers.Input(shape=(max_seq_length,), dtype=tf.int32, name="segment_ids") bert_layer = hub.KerasLayer(bert_path, trainable=True) pooled_output, sequence_output = bert_layer([input_word_ids, input_mask, segment_ids]) output = layers.Dense(1, activation='sigmoid')(pooled_output) model = tf.keras.models.Model(inputs=[input_word_ids, input_mask, segment_ids], outputs=output) return model # 准备数据 def create_input_data(sentences, labels): tokenizer = bert.tokenization.FullTokenizer(vocab_file=bert_path + "trainer/vocab.small", do_lower_case=True) # tokenizer = BertTokenizer.from_pretrained('bert-base-uncased') input_ids = [] input_masks = [] segment_ids = [] for sentence in sentences: tokens = tokenizer.tokenize(sentence) tokens = ["[CLS]"] + tokens + ["[SEP]"] input_id = tokenizer.convert_tokens_to_ids(tokens) input_mask = [1] * len(input_id) segment_id = [0] * len(input_id) padding_length = max_seq_length - len(input_id) input_id += [0] * padding_length input_mask += [0] * padding_length segment_id += [0] * padding_length input_ids.append(input_id) input_masks.append(input_mask) segment_ids.append(segment_id) return np.array(input_ids), np.array(input_masks), np.array(segment_ids), np.array(labels) # 加载训练数据 train_sentences = ["Example sentence 1", "Example sentence 2", ...] train_labels = [0, 1, ...] train_input_ids, train_input_masks, train_segment_ids, train_labels = create_input_data(train_sentences, train_labels) # 构建模型 model = create_model() model.compile(optimizer=tf.keras.optimizers.Adam(lr=learning_rate), loss='binary_crossentropy', metrics=['accuracy']) # 开始微调 model.fit([train_input_ids, train_input_masks, train_segment_ids], train_labels, batch_size=train_batch_size, epochs=num_train_epochs)
这段代码是用 TensorFlow 和 BERT 模型进行文本分类的示例。首先定义了模型路径和参数,然后使用 `hub.KerasLayer` 加载 BERT 模型,对输入进行编码后,添加一个全连接层并进行二分类,构建一个分类模型。接着使用 `bert.tokenization.FullTokenizer` 对输入数据进行编码,最后使用 `model.fit` 进行微调训练。这个示例并不是完整的代码,需要根据实际情况进行修改。
指出以下代码错误并修改。input_texts = [] target_texts = [] input_characters = set() target_characters = set() with open(data_path, 'r', encoding='utf-8') as f: lines = f.read().split('\n') for line in lines[: min(num_samples, len(lines) - 1)]: try: input_text,target_text = line.split('\t') target_text = '\t' + target_text + '\n' input_texts.append(input_text) target_texts.append(target_text) for char in input_text.strip(): if char not in input_characters: input_characters.add(char) for char in target_text.strip(): if char not in target_characters: target_characters.add(char) except ValueError: pass input_characters = sorted(list(input_characters)) target_characters = sorted(list(target_characters)) num_encoder_tokens = len(input_characters) num_decoder_tokens = len(target_characters) max_encoder_seq_length = max([len(txt) for txt in input_texts]) max_decoder_seq_length = max([len(txt) for txt in target_texts])
这段代码没有明显的语法错误,但是存在逻辑错误。如果数据集中有空行,代码可能会出错。此外,如果数据集中的行数小于 `num_samples`,代码也可能会出错。因此,我们应该在读取数据之前,先添加一个判断语句,确保数据集中的行数不小于 `num_samples`。
另外,在计算 `max_encoder_seq_length` 和 `max_decoder_seq_length` 时,应该使用 `strip()` 函数去掉每个行末尾的空格和换行符。
有关代码的修改建议如下:
```python
input_texts = []
target_texts = []
input_characters = set()
target_characters = set()
with open(data_path, 'r', encoding='utf-8') as f:
lines = f.read().split('\n')
if len(lines) < num_samples:
num_samples = len(lines) - 1
for line in lines[:num_samples]:
try:
input_text, target_text = line.split('\t')
target_text = '\t' + target_text + '\n'
input_texts.append(input_text)
target_texts.append(target_text)
for char in input_text.strip():
input_characters.add(char)
for char in target_text.strip():
target_characters.add(char)
except ValueError:
pass
input_characters = sorted(list(input_characters))
target_characters = sorted(list(target_characters))
num_encoder_tokens = len(input_characters)
num_decoder_tokens = len(target_characters)
max_encoder_seq_length = max([len(txt.strip()) for txt in input_texts])
max_decoder_seq_length = max([len(txt.strip()) for txt in target_texts])
```
修改后的代码中,我们添加了一个判断语句,确保读取的行数不会超过数据集的行数。同时,在计算序列的最大长度时,我们使用了 `strip()` 函数去掉每个行末尾的空格和换行符。
阅读全文
相关推荐
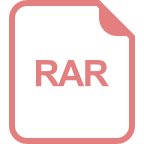
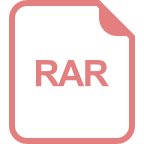
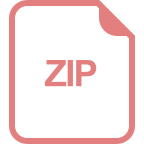



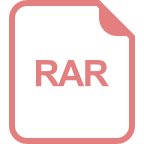
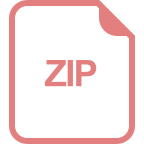
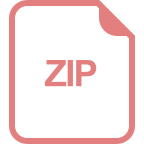
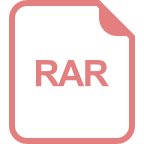
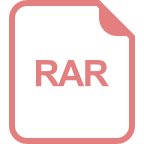
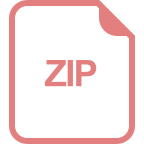
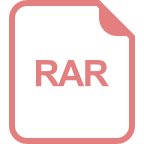
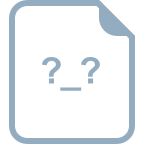
