tensor.argmax()如何使用
时间: 2023-05-24 20:05:45 浏览: 285
`torch.argmax()` 是 PyTorch 库中的一个函数,用于求一个张量(tensor)中的最大值所在的位置。具体使用方法如下:
1. 导入 PyTorch 库:
```
import torch
```
2. 创建一个张量:
```
x = torch.tensor([2, 7, 1, 9])
```
3. 求出张量 `x` 中最大值所在的位置:
```
idx = torch.argmax(x)
```
这里 `idx` 就是 `x` 中最大值所在的位置,也就是张量 `x` 中的第 3 个位置,因为 `x` 的第 3 个位置上的元素是 9。
如果张量是一个多维的张量,那么 `torch.argmax()` 可以在某个维度上求出最大值所在的位置。例如:
```
y = torch.tensor([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
idx = torch.argmax(y, dim=1)
```
这里 `dim=1` 表示要在每一行(即第 1 个维度)上求最大值所在的位置,所以 `idx` 的结果是 `[2, 2, 2]`,表示第 1 行最大值在第 3 列,第 2 行最大值在第 3 列,第 3 行最大值在第 3 列。
相关问题
np.argmax能用于tensor吗
可以,`np.argmax`可以用于numpy数组和tensor。如果你想在PyTorch中使用`argmax`函数,可以使用`torch.argmax`函数。例如,如果你有一个PyTorch张量`x`,并想找到它的最大值,你可以这样做:
```
import torch
x = torch.tensor([[1, 2, 3], [4, 5, 6]])
max_indices = torch.argmax(x)
print(max_indices) # 输出:tensor(5)
```
如果你想在TensorFlow中使用`argmax`函数,可以使用`tf.argmax`函数。例如,如果你有一个TensorFlow张量`x`,并想找到它的最大值,你可以这样做:
```
import tensorflow as tf
x = tf.constant([[1, 2, 3], [4, 5, 6]])
max_indices = tf.argmax(x)
print(max_indices) # 输出:tf.Tensor([1 1 1], shape=(3,), dtype=int64)
```
需要注意的是,这两个函数返回的最大值索引的形状可能是不同的,具体取决于输入张量的形状。
torch.argmax.
torch.argmax函数是一个PyTorch库中的函数,用于在指定维度上返回张量中最大值的索引。可以用它来实现分类任务中的预测值计算,以及模型评估中的准确率计算等等。其函数定义如下:
```
torch.argmax(input, dim=None, keepdim=False)
```
其中,input是需要计算最大值索引的输入张量,dim表示需要沿着哪个维度计算最大值索引(默认是整个张量),keepdim表示是否保持维度大小(默认是False)。
例如,对于一个2*3的矩阵tensor,可以使用如下代码计算每行的最大值以及对应的索引:
```
import torch
tensor = torch.tensor([[1, 2, 3], [4, 5, 6]])
max_val, max_idx = torch.max(tensor, dim=1)
print("max_val:", max_val) # 输出:max_val: tensor([3, 6])
print("max_idx:", max_idx) # 输出:max_idx: tensor([2, 2])
```
相关推荐
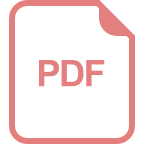
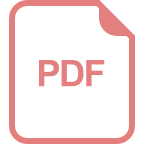
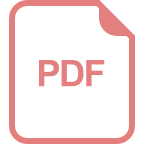












