用链表结构存储多项式,求两个多项式A+B的和,c语言代码,简洁款
时间: 2024-01-30 19:01:55 浏览: 80
好的,以下是一个简洁的 C 语言代码,可以用链表结构存储多项式,求两个多项式 A+B 的和:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义多项式项结构体
typedef struct PolyNode {
int coef; // 系数
int expon; // 指数
struct PolyNode *next; // 下一项
} PolyNode, *Polynomial;
// 创建一个新的多项式项
Polynomial createPolyNode(int coef, int expon) {
Polynomial node = (Polynomial)malloc(sizeof(PolyNode));
node->coef = coef;
node->expon = expon;
node->next = NULL;
return node;
}
// 将新的多项式项插入到多项式中
void insertPolyNode(Polynomial *p, Polynomial node) {
if (*p == NULL) {
*p = node;
} else if ((*p)->expon < node->expon) {
node->next = *p;
*p = node;
} else if ((*p)->expon == node->expon) {
(*p)->coef += node->coef;
free(node);
} else {
insertPolyNode(&((*p)->next), node);
}
}
// 读取多项式的每一项,并将它们插入到多项式中
Polynomial readPoly() {
int n, coef, expon;
scanf("%d", &n);
Polynomial poly = NULL;
while (n--) {
scanf("%d %d", &coef, &expon);
insertPolyNode(&poly, createPolyNode(coef, expon));
}
return poly;
}
// 计算两个多项式的和
Polynomial addPoly(Polynomial a, Polynomial b) {
Polynomial c = NULL;
while (a && b) {
if (a->expon > b->expon) {
insertPolyNode(&c, createPolyNode(a->coef, a->expon));
a = a->next;
} else if (a->expon < b->expon) {
insertPolyNode(&c, createPolyNode(b->coef, b->expon));
b = b->next;
} else {
int sum = a->coef + b->coef;
if (sum) {
insertPolyNode(&c, createPolyNode(sum, a->expon));
}
a = a->next;
b = b->next;
}
}
while (a) {
insertPolyNode(&c, createPolyNode(a->coef, a->expon));
a = a->next;
}
while (b) {
insertPolyNode(&c, createPolyNode(b->coef, b->expon));
b = b->next;
}
return c;
}
// 输出多项式的每一项
void printPoly(Polynomial poly) {
if (!poly) {
printf("0\n");
return;
}
while (poly) {
printf("%d %d", poly->coef, poly->expon);
poly = poly->next;
if (poly) {
printf(" ");
} else {
printf("\n");
}
}
}
int main() {
Polynomial a = readPoly();
Polynomial b = readPoly();
Polynomial c = addPoly(a, b);
printPoly(c);
return 0;
}
```
希望这个简洁的代码可以帮助到你!如果你有任何问题,请随时问我。
阅读全文
相关推荐
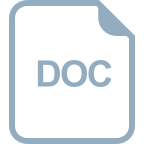
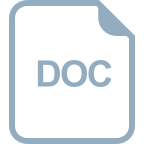
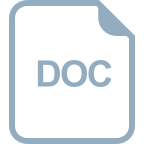





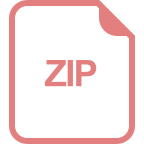









