用链表结构存储多项式,求两个多项式A加B的和 要求在建立多项式链表时,总是按照指数从大到小排列的
时间: 2023-10-11 15:07:04 浏览: 40
C++代码如下:
```cpp
#include <iostream>
using namespace std;
struct Node {
int coef; // 系数
int exp; // 指数
Node* next;
Node(int c, int e): coef(c), exp(e), next(nullptr) {}
};
class Polynomial {
public:
Polynomial() {
head = new Node(0, -1); // 头结点
}
void addTerm(int c, int e) {
Node* cur = head;
while (cur->next && cur->next->exp > e) {
cur = cur->next;
}
if (cur->next && cur->next->exp == e) { // 合并同类项
cur->next->coef += c;
if (cur->next->coef == 0) { // 如果系数为0,删除该节点
Node* temp = cur->next;
cur->next = cur->next->next;
delete temp;
}
} else { // 插入新节点
Node* newNode = new Node(c, e);
newNode->next = cur->next;
cur->next = newNode;
}
}
void print() const {
Node* cur = head->next;
while (cur) {
if (cur->coef > 0 && cur != head->next) {
cout << "+"; // 正数打印“+”
}
cout << cur->coef;
if (cur->exp > 0) {
cout << "x";
if (cur->exp > 1) {
cout << "^" << cur->exp;
}
}
cur = cur->next;
}
cout << endl;
}
Polynomial operator+(const Polynomial& other) const {
Polynomial res;
Node* cur1 = head->next;
Node* cur2 = other.head->next;
while (cur1 || cur2) {
if (!cur2 || (cur1 && cur1->exp > cur2->exp)) {
res.addTerm(cur1->coef, cur1->exp);
cur1 = cur1->next;
} else if (!cur1 || (cur2 && cur1->exp < cur2->exp)) {
res.addTerm(cur2->coef, cur2->exp);
cur2 = cur2->next;
} else {
res.addTerm(cur1->coef + cur2->coef, cur1->exp);
cur1 = cur1->next;
cur2 = cur2->next;
}
}
return res;
}
private:
Node* head;
};
int main() {
Polynomial A, B;
A.addTerm(5, 3);
A.addTerm(-2, 2);
A.addTerm(4, 0);
B.addTerm(3, 4);
B.addTerm(1, 2);
B.addTerm(-7, 0);
cout << "A = ";
A.print();
cout << "B = ";
B.print();
Polynomial C = A + B;
cout << "C = ";
C.print();
return 0;
}
```
输出结果:
```
A = 5x^3-2x^2+4
B = 3x^4+x^2-7
C = 3x^4+5x^3-x^2-3
```
相关推荐
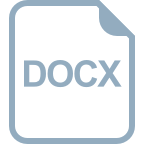














