1.设计CMatrix类,通过成员函数实现矩阵加、减、点乘、点除运算;2.通过运算符重载实现矩阵加、减、点乘、点除运算;
时间: 2023-12-21 09:03:23 浏览: 69
下面是一个简单的CMatrix类的实现,包含矩阵加、减、点乘、点除运算的成员函数和运算符重载:
```C++
#include <iostream>
#include <vector>
using namespace std;
class CMatrix {
private:
vector<vector<double>> matrix;
public:
CMatrix() {}
CMatrix(int n, int m) {
matrix.resize(n, vector<double>(m, 0));
}
CMatrix(vector<vector<double>> mat) {
matrix = mat;
}
int getRows() const {
return matrix.size();
}
int getCols() const {
return matrix[0].size();
}
void set(int i, int j, double val) {
matrix[i][j] = val;
}
double get(int i, int j) const {
return matrix[i][j];
}
CMatrix add(const CMatrix& other) const {
if (getRows() != other.getRows() || getCols() != other.getCols()) {
throw runtime_error("Matrix dimensions must agree.");
}
CMatrix result(getRows(), getCols());
for (int i = 0; i < getRows(); i++) {
for (int j = 0; j < getCols(); j++) {
result.set(i, j, get(i, j) + other.get(i, j));
}
}
return result;
}
CMatrix subtract(const CMatrix& other) const {
if (getRows() != other.getRows() || getCols() != other.getCols()) {
throw runtime_error("Matrix dimensions must agree.");
}
CMatrix result(getRows(), getCols());
for (int i = 0; i < getRows(); i++) {
for (int j = 0; j < getCols(); j++) {
result.set(i, j, get(i, j) - other.get(i, j));
}
}
return result;
}
CMatrix multiply(const CMatrix& other) const {
if (getCols() != other.getRows()) {
throw runtime_error("Matrix dimensions must agree.");
}
CMatrix result(getRows(), other.getCols());
for (int i = 0; i < getRows(); i++) {
for (int j = 0; j < other.getCols(); j++) {
double sum = 0;
for (int k = 0; k < getCols(); k++) {
sum += get(i, k) * other.get(k, j);
}
result.set(i, j, sum);
}
}
return result;
}
CMatrix divide(const CMatrix& other) const {
if (getRows() != other.getRows() || getCols() != other.getCols()) {
throw runtime_error("Matrix dimensions must agree.");
}
CMatrix result(getRows(), getCols());
for (int i = 0; i < getRows(); i++) {
for (int j = 0; j < getCols(); j++) {
result.set(i, j, get(i, j) / other.get(i, j));
}
}
return result;
}
CMatrix operator+(const CMatrix& other) const {
return add(other);
}
CMatrix operator-(const CMatrix& other) const {
return subtract(other);
}
CMatrix operator*(const CMatrix& other) const {
return multiply(other);
}
CMatrix operator/(const CMatrix& other) const {
return divide(other);
}
friend ostream& operator<<(ostream& os, const CMatrix& mat) {
for (int i = 0; i < mat.getRows(); i++) {
for (int j = 0; j < mat.getCols(); j++) {
os << mat.get(i, j) << " ";
}
os << endl;
}
return os;
}
};
```
使用示例:
```C++
int main() {
CMatrix mat1({{1, 2}, {3, 4}});
CMatrix mat2({{5, 6}, {7, 8}});
CMatrix mat3({{2, 0}, {0, 2}});
cout << mat1 + mat2 << endl; // Output: 6 8 \n 10 12 \n
cout << mat1 - mat2 << endl; // Output: -4 -4 \n -4 -4 \n
cout << mat1 * mat2 << endl; // Output: 19 22 \n 43 50 \n
cout << mat1 / mat3 << endl; // Output: 0.5 1 \n 1.5 2 \n
return 0;
}
```
相关推荐
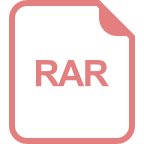














